def data_processing(data): # 日期缺失,补充 data.fillna(method='ffill', inplace=True) date_history = pd.DataFrame(data.iloc[:, 0]) data_history = pd.DataFrame(data.iloc[:, 1]) date_history = np.array(date_history) data_history = [x for item in np.array(data_history).tolist() for x in item] # 缺失值处理 history_time_list = [] for date in date_history: date_obj = datetime.datetime.strptime(date[0], '%Y/%m/%d %H:%M') #将字符串转为 datetime 对象 history_time_list.append(date_obj) start_time = history_time_list[0] # 起始时间 end_time = history_time_list[-1] # 结束时间 delta = datetime.timedelta(minutes=15) #时间间隔为15分钟 time_new_list = [] current_time = start_time while current_time <= end_time: time_new_list.append(current_time) current_time += delta # 缺失位置记录 code_list = [] for i in range(len(time_new_list)): code_list = code_list history_time_list = history_time_list while (time_new_list[i] - history_time_list[i]) != datetime.timedelta(minutes=0): history_time_list.insert(i, time_new_list[i]) code_list.append(i) for i in code_list: data_history.insert(i, data_history[i - 1]) # 输出补充好之后的数据 data = pd.DataFrame({'date': time_new_list, 'load': data_history}) return data 代码 优化
时间: 2024-01-11 13:01:59 浏览: 24
这段代码可以进行以下优化:
1. 将日期转换为 datetime 对象的操作可以放在读取数据时进行,避免在处理数据时重复执行。
2. 用 pandas 的 resample 函数可以方便地实现时间序列的重采样。可以先将日期列设置为索引,然后使用 resample 函数按照指定的时间间隔进行重采样,再使用 ffill 方法填充缺失值。
3. 可以使用 pandas 的 merge 函数将重采样之后的数据与原数据进行合并,这样可以避免使用循环操作。
下面是优化后的代码:
```
import pandas as pd
import datetime
def data_processing(data):
# 将日期转换为 datetime 对象
data['date'] = pd.to_datetime(data['date'], format='%Y/%m/%d %H:%M')
data.set_index('date', inplace=True)
# 重采样,补全缺失值
data_resampled = data.resample('15T').ffill()
# 合并原数据和重采样之后的数据
data_processed = data_resampled.merge(data, how='outer', left_index=True, right_index=True)
return data_processed
```
相关问题
data 是datafame格式,def data_processing(data): # 日期缺失,补充 data.fillna(method='ffill', inplace=True) date_history = pd.DataFrame(data.iloc[:, 0]) data_history = pd.DataFrame(data.iloc[:, 1]) date_history = np.array(date_history) data_history = [x for item in np.array(data_history).tolist() for x in item] # 缺失值处理 history_time_list = [] for date in date_history: date_obj = datetime.datetime.strptime(date[0], '%Y/%m/%d %H:%M') #将字符串转为 datetime 对象 history_time_list.append(date_obj) start_time = history_time_list[0] # 起始时间 end_time = history_time_list[-1] # 结束时间 delta = datetime.timedelta(minutes=15) #时间间隔为15分钟 time_new_list = [] current_time = start_time while current_time <= end_time: time_new_list.append(current_time) current_time += delta # 缺失位置记录 code_list = [] for i in range(len(time_new_list)): code_list = code_list history_time_list = history_time_list while (time_new_list[i] - history_time_list[i]) != datetime.timedelta(minutes=0): history_time_list.insert(i, time_new_list[i]) code_list.append(i) for i in code_list: data_history.insert(i, data_history[i - 1]) # 输出补充好之后的数据 data = pd.DataFrame({'date': time_new_list, 'load': data_history}) return data 优化代码
可以优化的部分如下:
1. 将 date_history 和 data_history 的赋值语句合并为一行,即 `date_history, data_history = data.iloc[:, :2].values.T`。
2. 不需要将 date_history 转换为 numpy array,因为 iloc 输出的已经是 numpy array 类型了。
3. 在处理日期缺失时,可以使用 pandas 的 resample 函数来实现时间间隔的补充,避免手动循环。
4. 在处理缺失位置时,可以使用 pandas 的 interpolate 函数来进行插值。
优化后的代码如下所示:
```python
def data_processing(data):
# 日期缺失,补充
data.fillna(method='ffill', inplace=True)
date_history, data_history = data.iloc[:, :2].values.T
# 转换为 datetime 对象
date_history = pd.to_datetime(date_history, format='%Y/%m/%d %H:%M')
# 时间间隔为15分钟,使用 resample 补充缺失数据
data = pd.DataFrame({'load': data_history}, index=date_history)
data = data.resample('15T').ffill()
# 使用 interpolate 函数进行插值
data['load'] = data['load'].interpolate()
# 输出补充好之后的数据
data.reset_index(inplace=True)
data.rename(columns={'index': 'date'}, inplace=True)
return data
```
class LinkNode: #单链表结点类 def __init__(self,data=None): #构造函数 self.data=data #data属性 self.next=None #next属性 class LinkList: #单链表类 def __init__(self): #构造函数 self.head=LinkNode() #头结点head self.head.next=None def CreateListF(self, a): #头插法:由数组a整体建立单链表 def CreateListR(self, a): #尾插法:由数组a整体建立单链表 def geti(self, i): #返回序号为i的结点 p=self.head j=-1 while (j<i and p is not None): j+=1 p=p.next return p def Add(self, e): #在线性表的末尾添加一个元素e def getsize(self): #返回长度 p=self.head cnt=0 while p.next is not None: #找到尾结点为止 cnt+=1 p=p.next return cnt
好的,那么接下来我会帮助您补齐这段代码的缺失部分。请注意,以下代码仅供参考,具体实现可能因情况而异,您可以根据自己的需求进行修改:
```python
class LinkNode: #单链表结点类
def __init__(self,data=None): #构造函数
self.data=data #data属性
self.next=None #next属性
class LinkList: #单链表类
def __init__(self): #构造函数
self.head=LinkNode() #头结点head
self.head.next=None
def CreateListF(self, a): #头插法:由数组a整体建立单链表
for i in range(len(a)):
node = LinkNode(a[i])
node.next = self.head.next
self.head.next = node
def CreateListR(self, a): #尾插法:由数组a整体建立单链表
p = self.head
for i in range(len(a)):
node = LinkNode(a[i])
p.next = node
p = node
def geti(self, i): #返回序号为i的结点
p = self.head
j = -1
while (j < i and p is not None):
j += 1
p = p.next
return p
def Add(self, e): #在线性表的末尾添加一个元素e
p = self.head
while p.next is not None:
p = p.next
node = LinkNode(e)
p.next = node
def getsize(self): #返回长度
p = self.head
cnt = 0
while p.next is not None: #找到尾结点为止
cnt += 1
p = p.next
return cnt
```
相关推荐
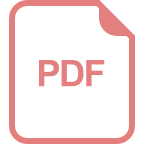
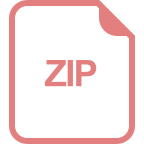
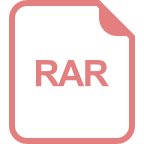













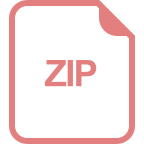