写一个烟花代码Python
时间: 2023-11-21 08:58:16 浏览: 140
下面是一个简单的 Python 烟花代码,使用 Pygame 模块:
```python
import pygame
import random
# 初始化 Pygame
pygame.init()
# 定义屏幕大小
screen = pygame.display.set_mode((800, 600))
# 定义颜色
colors = [(255, 0, 0), (0, 255, 0), (0, 0, 255), (255, 255, 0), (255, 0, 255), (0, 255, 255)]
# 定义烟花类
class Firework:
def __init__(self, x, y):
self.x = x
self.y = y
self.color = random.choice(colors)
self.speed = 2
self.radius = 2
self.particles = []
self.life = 100
# 生成颗粒
for i in range(50):
angle = random.uniform(0, 2 * 3.14)
speed = random.uniform(1, 5)
self.particles.append([angle, speed])
# 更新颗粒位置
def update_particles(self):
for i in range(len(self.particles)):
angle, speed = self.particles[i]
self.particles[i][0] += 0.05
x = int(self.x + speed * self.radius * math.cos(angle))
y = int(self.y + speed * self.radius * math.sin(angle))
self.particles[i][1] -= 0.1
self.particles[i][1] = max(0, self.particles[i][1])
self.particles[i][2] = (x, y)
# 更新烟花位置
def update(self):
self.y -= self.speed
self.speed -= 0.1
self.radius += 0.1
self.life -= 1
if self.life <= 0:
return False
self.update_particles()
return True
# 绘制烟花
def draw(self):
pygame.draw.circle(screen, self.color, (int(self.x), int(self.y)), int(self.radius))
for particle in self.particles:
x, y = particle[2]
pygame.draw.circle(screen, self.color, (int(x), int(y)), 2)
# 存储所有烟花
fireworks = []
# 循环
clock = pygame.time.Clock()
done = False
while not done:
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
done = True
# 随机生成烟花
if random.random() < 0.1:
fireworks.append(Firework(random.uniform(0, 800), 600))
# 更新烟花
for firework in fireworks:
if not firework.update():
fireworks.remove(firework)
# 绘制烟花
screen.fill((0, 0, 0))
for firework in fireworks:
firework.draw()
# 更新屏幕
pygame.display.flip()
clock.tick(60)
# 退出 Pygame
pygame.quit()
```
运行代码后,你会看到屏幕上不断出现烟花,并且每个烟花都会爆炸成许多小颗粒。
阅读全文
相关推荐
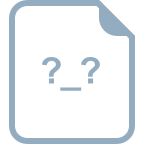
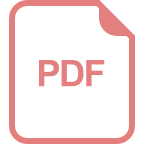






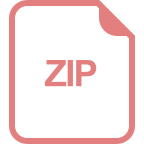
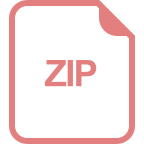
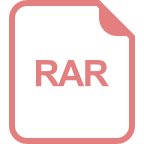
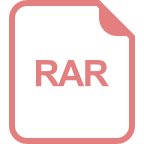
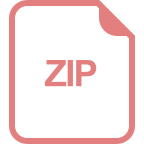
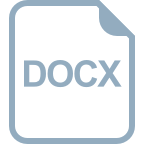