C语言类型
时间: 2023-09-06 18:11:28 浏览: 46
好的,以下是一个C语言版本的统计二叉树中度为1节点的代码示例:
```c
#include <stdio.h>
#include <stdlib.h>
struct TreeNode {
int val;
struct TreeNode* left;
struct TreeNode* right;
};
int countDegreeOne(struct TreeNode* root) {
if (root == NULL) {
return 0;
}
int count = 0;
if ((root->left != NULL && root->right == NULL) || (root->left == NULL && root->right != NULL)) {
count++;
}
count += countDegreeOne(root->left);
count += countDegreeOne(root->right);
return count;
}
int main() {
struct TreeNode* root = (struct TreeNode*)malloc(sizeof(struct TreeNode));
root->val = 1;
struct TreeNode* node1 = (struct TreeNode*)malloc(sizeof(struct TreeNode));
node1->val = 2;
struct TreeNode* node2 = (struct TreeNode*)malloc(sizeof(struct TreeNode));
node2->val = 3;
struct TreeNode* node3 = (struct TreeNode*)malloc(sizeof(struct TreeNode));
node3->val = 4;
struct TreeNode* node4 = (struct TreeNode*)malloc(sizeof(struct TreeNode));
node4->val = 5;
root->left = node1;
root->right = node2;
node1->left = node3;
node2->right = node4;
int count = countDegreeOne(root);
printf("The count of degree one node is %d", count);
free(root);
free(node1);
free(node2);
free(node3);
free(node4);
return 0;
}
```
在这个示例中,我们定义了一个 TreeNode 结构体来表示二叉树节点,并实现了一个 countDegreeOne 函数来求解度为1的节点数。在 countDegreeOne 函数中,我们首先对空节点进行了判断,然后判断当前节点的度是否为1,如果是,则计数器加1。最后递归遍历左右子节点,并将其子节点的度为1的节点数加到计数器中,最终返回计数器的值。在 main 函数中,我们创建了一个二叉树,并调用 countDegreeOne 函数求解度为1的节点数,并输出结果。最后释放申请的内存。
相关推荐
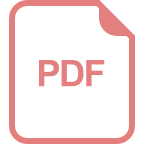
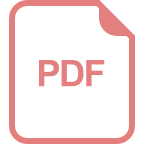















