用C++写一个程序包含1.类的定义,构造函数,成员函数 2.两个复数运算的运算符重载.重载 +、-、*、/、++、--、<<、>>等运算符 3. double型数可以转换成复数
时间: 2023-07-10 11:30:03 浏览: 54
好的,以下是一个符合您要求的 C++ 程序示例:
```cpp
#include <iostream>
using namespace std;
class Complex {
private:
double real; // 复数的实部
double imag; // 复数的虚部
public:
// 构造函数
Complex(double r = 0, double i = 0) : real(r), imag(i) {}
// 成员函数
double getReal() const { return real; }
double getImag() const { return imag; }
void setReal(double r) { real = r; }
void setImag(double i) { imag = i; }
// 运算符重载
Complex operator+(const Complex& c) const { return Complex(real + c.real, imag + c.imag); }
Complex operator-(const Complex& c) const { return Complex(real - c.real, imag - c.imag); }
Complex operator*(const Complex& c) const { return Complex(real * c.real - imag * c.imag, real * c.imag + imag * c.real); }
Complex operator/(const Complex& c) const {
double denominator = c.real * c.real + c.imag * c.imag;
return Complex((real * c.real + imag * c.imag) / denominator, (imag * c.real - real * c.imag) / denominator);
}
Complex operator++() { return Complex(++real, ++imag); }
Complex operator++(int) { return Complex(real++, imag++); }
Complex operator--() { return Complex(--real, --imag); }
Complex operator--(int) { return Complex(real--, imag--); }
friend ostream& operator<<(ostream& os, const Complex& c);
friend istream& operator>>(istream& is, Complex& c);
};
// 友元函数,重载输出运算符
ostream& operator<<(ostream& os, const Complex& c) {
os << c.real << "+" << c.imag << "i";
return os;
}
// 友元函数,重载输入运算符
istream& operator>>(istream& is, Complex& c) {
is >> c.real >> c.imag;
return is;
}
// 将 double 类型转换成复数类型
inline Complex doubleToComplex(double x) {
return Complex(x, 0);
}
int main() {
Complex c1(1, 2), c2(3, 4);
cout << "c1 = " << c1 << endl;
cout << "c2 = " << c2 << endl;
cout << "c1 + c2 = " << c1 + c2 << endl;
cout << "c1 - c2 = " << c1 - c2 << endl;
cout << "c1 * c2 = " << c1 * c2 << endl;
cout << "c1 / c2 = " << c1 / c2 << endl;
cout << "++c1 = " << ++c1 << endl;
cout << "--c2 = " << --c2 << endl;
double d = 5.6;
Complex c3 = doubleToComplex(d);
cout << "c3 = " << c3 << endl;
return 0;
}
```
该程序中,`Complex` 类表示复数,包含实部和虚部两个成员变量,以及相应的构造函数和成员函数。该类还重载了加减乘除、自增自减、输入输出运算符,并提供了将 `double` 类型转换成 `Complex` 类型的函数。在 `main` 函数中,我们创建了两个复数对象 `c1` 和 `c2`,并进行了加减乘除以及自增自减等操作。同时,我们还将一个 `double` 类型的变量转换成了一个复数对象 `c3`。
相关推荐
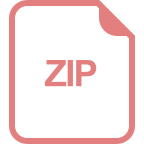
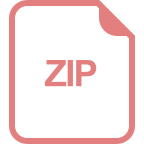
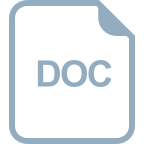














