AOP面向切面编程的测试代码以及依赖导入
时间: 2023-10-30 08:48:51 浏览: 131
以下是一个使用Spring框架实现AOP面向切面编程的测试代码示例。
首先,创建一个切面类 `LoggingAspect`,用于定义切面逻辑:
```java
import org.aspectj.lang.JoinPoint;
import org.aspectj.lang.annotation.After;
import org.aspectj.lang.annotation.Aspect;
import org.aspectj.lang.annotation.Before;
import org.springframework.stereotype.Component;
@Aspect
@Component
public class LoggingAspect {
@Before("execution(* com.example.service.*.*(..))")
public void beforeAdvice(JoinPoint joinPoint) {
System.out.println("Before method: " + joinPoint.getSignature().getName());
}
@After("execution(* com.example.service.*.*(..))")
public void afterAdvice(JoinPoint joinPoint) {
System.out.println("After method: " + joinPoint.getSignature().getName());
}
}
```
然后,创建一个测试服务类 `UserService`,用于演示AOP的应用:
```java
import org.springframework.stereotype.Service;
@Service
public class UserService {
public void createUser(String username) {
System.out.println("Creating user: " + username);
}
public void deleteUser(String username) {
System.out.println("Deleting user: " + username);
}
}
```
最后,创建一个Spring Boot应用程序,并在启动类中进行配置:
```java
import org.springframework.boot.SpringApplication;
import org.springframework.boot.autoconfigure.SpringBootApplication;
import org.springframework.context.annotation.EnableAspectJAutoProxy;
@SpringBootApplication
@EnableAspectJAutoProxy
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
UserService userService = context.getBean(UserService.class);
userService.createUser("John");
userService.deleteUser("John");
}
}
```
在上述示例中,`LoggingAspect` 切面类使用 `@Before` 和 `@After` 注解分别定义了在目标方法执行前和执行后的逻辑。切面逻辑会应用于 `UserService` 类中的所有方法。
为了能够运行Spring Boot应用程序,需要在项目的构建工具(如Maven或Gradle)的配置文件中添加Spring相关的依赖。
如果使用Maven,可以在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter</artifactId>
<version>2.5.3</version>
</dependency>
```
如果使用Gradle,可以在build.gradle文件中添加以下依赖:
```groovy
implementation 'org.springframework.boot:spring-boot-starter:2.5.3'
```
这样,就完成了AOP面向切面编程的测试代码的实现和依赖导入。运行应用程序时,可以看到切面逻辑在方法执行前和执行后打印了相应的日志消息。
阅读全文
相关推荐
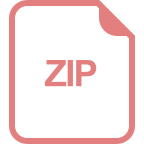
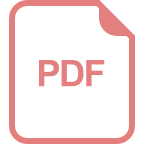
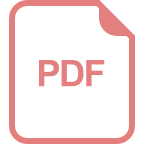
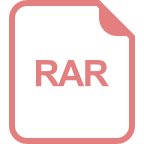
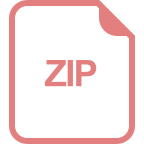
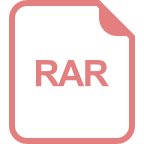
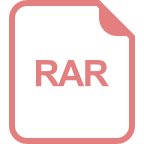
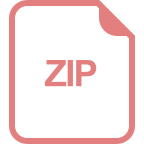
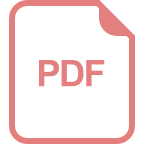
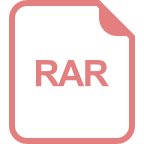
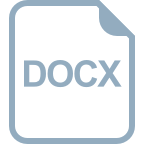
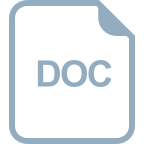
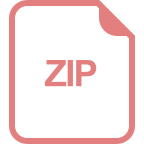
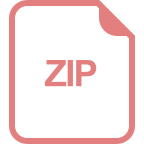
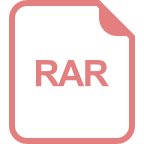
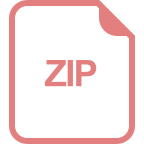