用canvas写个坦克大战
时间: 2023-06-22 12:36:59 浏览: 48
以下是一个简单的坦克大战游戏的示例代码:
```html
<!DOCTYPE html>
<html>
<head>
<title>坦克大战</title>
<style>
canvas {
border: 1px solid black;
}
</style>
</head>
<body>
<canvas id="gameCanvas" width="480" height="320"></canvas>
<script>
// 获取画布和上下文
var canvas = document.getElementById("gameCanvas");
var ctx = canvas.getContext("2d");
// 定义坦克
var tank = {
x: canvas.width / 2,
y: canvas.height - 50,
width: 50,
height: 50,
color: "green",
speed: 5,
direction: "right"
};
// 定义子弹
var bullets = [];
var bulletWidth = 10;
var bulletHeight = 20;
var bulletSpeed = 10;
// 监听方向键按下事件,控制坦克移动和发射子弹
document.addEventListener("keydown", function(event) {
if (event.keyCode === 37) { // 左
tank.direction = "left";
tank.x -= tank.speed;
} else if (event.keyCode === 39) { // 右
tank.direction = "right";
tank.x += tank.speed;
} else if (event.keyCode === 32) { // 空格
bullets.push({
x: tank.x + tank.width / 2 - bulletWidth / 2,
y: tank.y - bulletHeight,
width: bulletWidth,
height: bulletHeight
});
}
});
// 游戏循环
function gameLoop() {
// 清空画布
ctx.clearRect(0, 0, canvas.width, canvas.height);
// 绘制坦克
ctx.fillStyle = tank.color;
ctx.fillRect(tank.x, tank.y, tank.width, tank.height);
// 移动子弹
for (var i = 0; i < bullets.length; i++) {
if (bullets[i].y < 0) {
bullets.splice(i, 1);
i--;
} else {
bullets[i].y -= bulletSpeed;
ctx.fillStyle = "black";
ctx.fillRect(bullets[i].x, bullets[i].y, bullets[i].width, bullets[i].height);
}
}
// 检测子弹是否击中坦克
for (var i = 0; i < bullets.length; i++) {
if (bullets[i].x < tank.x + tank.width && bullets[i].x + bullets[i].width > tank.x
&& bullets[i].y < tank.y + tank.height && bullets[i].y + bullets[i].height > tank.y) {
alert("Game Over!");
location.reload();
}
}
// 循环调用游戏循环
requestAnimationFrame(gameLoop);
}
// 开始游戏循环
gameLoop();
</script>
</body>
</html>
```
这段代码使用canvas绘制了一个简单的坦克大战游戏,您可以在浏览器中打开并运行它来查看效果。在游戏中,玩家可以通过按下左右方向键来控制坦克的移动方向,按下空格键来发射子弹。如果子弹击中坦克,则游戏结束。
相关推荐
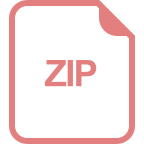









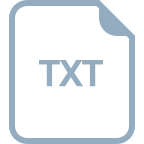
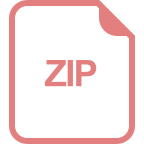
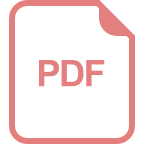