c语言写代码 将结构体输出json字符串,并保存成文件
时间: 2024-01-12 20:05:21 浏览: 27
好的,下面是一个示例代码,将结构体输出为JSON字符串并保存到文件中:
```c
#include <stdio.h>
#include <stdlib.h>
#include <json-c/json.h>
// 定义一个结构体
typedef struct {
char name[20];
int age;
char email[30];
} Person;
int main() {
// 初始化结构体变量
Person p = {"张三", 25, "zhangsan@example.com"};
// 创建JSON对象
json_object *jobj = json_object_new_object();
json_object *jname = json_object_new_string(p.name);
json_object *jage = json_object_new_int(p.age);
json_object *jemail = json_object_new_string(p.email);
// 将结构体成员添加到JSON对象中
json_object_object_add(jobj, "name", jname);
json_object_object_add(jobj, "age", jage);
json_object_object_add(jobj, "email", jemail);
// 将JSON对象转换为字符串
const char *json_string = json_object_to_json_string(jobj);
// 将字符串保存到文件中
FILE *fp = fopen("person.json", "w");
if (fp == NULL) {
fprintf(stderr, "无法打开文件\n");
exit(1);
}
fprintf(fp, "%s", json_string);
fclose(fp);
// 释放JSON对象
json_object_put(jobj);
return 0;
}
```
在这个例子中,我们使用了JSON-C库中的json_object_new_object()函数来创建一个JSON对象。然后,我们使用json_object_new_string()和json_object_new_int()函数分别创建字符串类型和整数类型的JSON值,并将它们添加到JSON对象中。最后,我们使用json_object_to_json_string()函数将JSON对象转换为字符串,并将其保存到文件中。注意,我们还需要使用json_object_put()函数释放JSON对象的内存。
相关推荐
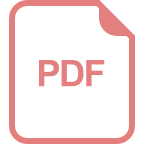
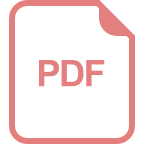














