pyqt5 qmessagebox定时关闭
时间: 2023-05-02 12:04:19 浏览: 173
使用PyQt5的QMessageBox能够实现消息提示框,也能够设置自动关闭功能。使用QMessageBox的setStandardButtons函数可以设置提示框的按钮,使用QMessageBox的setWindowModality函数可以使提示框成为模态窗口。而使用QTimer的singleShot函数可以实现定时器效果,从而设置自动关闭功能。具体使用方法可以参考PyQt5官方文档和相关示例代码。
相关问题
pyqt5 qmessagebox
QMessageBox is a class in PyQt5 that provides a simple way to display message boxes. These message boxes can be used to display information to the user or to get input from the user.
To use QMessageBox in PyQt5, you first need to import it:
```python
from PyQt5.QtWidgets import QMessageBox
```
Once you have imported QMessageBox, you can create an instance of it and set its properties. Here's an example:
```python
msg_box = QMessageBox()
msg_box.setText("Hello, world!")
msg_box.setWindowTitle("My Message Box")
msg_box.setIcon(QMessageBox.Information)
msg_box.setStandardButtons(QMessageBox.Ok | QMessageBox.Cancel)
msg_box.setDefaultButton(QMessageBox.Ok)
```
In this example, we create a message box with the text "Hello, world!", a title of "My Message Box", an information icon, and two standard buttons (Ok and Cancel). We also set the default button to be the Ok button.
To display the message box, you can call its exec() method:
```python
result = msg_box.exec_()
```
This will display the message box and wait for the user to interact with it. When the user clicks a button, the exec() method will return a value indicating which button was clicked. In this example, we store the result in the variable result.
Here's an example that shows how to use QMessageBox to get input from the user:
```python
name, ok_pressed = QMessageBox.getText(
None, "Get Name", "Enter your name:")
if ok_pressed:
print("Hello, " + name)
```
In this example, we use the static method getText() to display a message box with a text input field. We pass in the parent widget (None), a title of "Get Name", and a message of "Enter your name:". The method returns two values: the text entered by the user and a boolean indicating whether the Ok button was pressed. If the Ok button was pressed, we print a greeting to the console.
pyqt5 QMessageBox
PyQt5的QMessageBox是一种通用的弹出框,用于显示消息、警告、错误、提问等等。它可以在图形用户界面中与用户进行交互,并根据用户的选择执行相应的操作。
在PyQt5中使用QMessageBox可以显示不同类型的消息框,并且可以自定义按钮的文本。例如,使用QMessageBox的information方法可以显示一个信息框,question方法可以显示一个问答框,warning方法可以显示一个警告框,critical方法可以显示一个危险框,about方法可以显示一个关于框。
为了让这些按钮显示我们喜欢的中文,可以通过以下两种方式实现:
方式一:使用setButtonText方法设置按钮的文本。例如,使用QMessageBox的setButtonText方法可以设置Yes按钮的文本为"确定",No按钮的文本为"取消"。
方式二:在创建QMessageBox对象时,可以指定按钮的文本。例如,使用QMessageBox的question方法创建一个问答框时,可以将按钮的文本设置为中文。
下面是一个使用PyQt5的QMessageBox的示例代码:
```python
from PyQt5.QtWidgets import QMessageBox, QWidget, QApplication
app = QApplication([])
# 创建一个QWidget作为父类窗口
w = QWidget()
# 显示一个退出确定框
reply = QMessageBox.question(w, '退出', '确定退出?', QMessageBox.Yes | QMessageBox.No | QMessageBox.Cancel, QMessageBox.Cancel)
if reply == QMessageBox.Yes:
print('退出')
app.quit()
else:
print('不退出')
app.exec_()
```
以上代码创建了一个QWidget对象作为父类窗口,然后使用QMessageBox的question方法显示一个问答框,用户可以选择是、否或取消。根据用户的选择,程序会打印相应的输出并退出或继续运行。
希望以上解答能帮到你。如果还有其他问题,请随时提问。<span class="em">1</span><span class="em">2</span><span class="em">3</span>
#### 引用[.reference_title]
- *1* [Python——pyqt5——消息框(QMessageBox)](https://blog.csdn.net/weixin_30254435/article/details/95785993)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 33.333333333333336%"]
- *2* [Pyqt5如何让QMessageBox按钮显示中文示例代码](https://download.csdn.net/download/weixin_38546817/14869947)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 33.333333333333336%"]
- *3* [python界面交互PyQt5–消息框(QMessageBox)](https://blog.csdn.net/qq_41551450/article/details/104947551)[target="_blank" data-report-click={"spm":"1018.2226.3001.9630","extra":{"utm_source":"vip_chatgpt_common_search_pc_result","utm_medium":"distribute.pc_search_result.none-task-cask-2~all~insert_cask~default-1-null.142^v93^chatsearchT3_1"}}] [.reference_item style="max-width: 33.333333333333336%"]
[ .reference_list ]
阅读全文
相关推荐
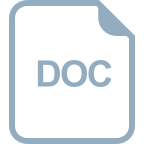
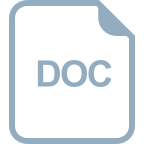
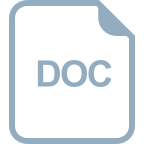













