SpringBoot 整合 MyBatis
时间: 2024-01-21 18:04:00 浏览: 31
在 Spring Boot 中整合 MyBatis,可以通过以下步骤实现:
1. 引入 MyBatis 和 MyBatis-Spring 的依赖。可以使用 Maven 或 Gradle 等构建工具,在 pom.xml 或 build.gradle 文件中添加以下依赖:
```
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis</artifactId>
<version>3.5.6</version>
</dependency>
<dependency>
<groupId>org.mybatis</groupId>
<artifactId>mybatis-spring</artifactId>
<version>2.0.6</version>
</dependency>
```
2. 配置数据源。可以在 application.properties 或 application.yml 文件中添加数据源的配置,例如:
```
spring.datasource.url=jdbc:mysql://localhost:3306/mydb
spring.datasource.username=root
spring.datasource.password=root
spring.datasource.driver-class-name=com.mysql.cj.jdbc.Driver
```
3. 配置 MyBatis。可以在 application.properties 或 application.yml 文件中添加 MyBatis 的配置,例如:
```
mybatis.mapper-locations=classpath:mapper/*.xml
mybatis.type-aliases-package=com.example.model
mybatis.configuration.map-underscore-to-camel-case=true
```
这里的 mapper-locations 是 mapper 文件的路径,type-aliases-package 是实体类的包名,configuration.map-underscore-to-camel-case 是开启下划线转驼峰命名规则。
4. 编写 Mapper 接口和 XML 文件。可以使用 @Mapper 注解标注 Mapper 接口,例如:
```
@Mapper
public interface UserMapper {
User selectUserById(Integer id);
}
```
然后在 resources 目录下创建 mapper 目录,编写对应的 XML 文件,例如:
```
<?xml version="1.0" encoding="UTF-8" ?>
<!DOCTYPE mapper PUBLIC "-//mybatis.org//DTD Mapper 3.0//EN" "http://mybatis.org/dtd/mybatis-3-mapper.dtd">
<mapper namespace="com.example.mapper.UserMapper">
<select id="selectUserById" parameterType="java.lang.Integer" resultType="com.example.model.User">
select * from user where id = #{id}
</select>
</mapper>
```
5. 在 Service 中使用 Mapper 接口。可以使用 @Autowired 注解注入 Mapper 接口,例如:
```
@Service
public class UserService {
@Autowired
private UserMapper userMapper;
public User getUserById(Integer id) {
return userMapper.selectUserById(id);
}
}
```
这样就可以在 Service 中使用 MyBatis 的 Mapper 接口了。
以上就是在 Spring Boot 中整合 MyBatis 的基本步骤。需要注意的是,如果使用的是 Spring Boot 2.x 版本,需要将 @MapperScan 注解加到启动类上,扫描 Mapper 接口。例如:
```
@SpringBootApplication
@MapperScan("com.example.mapper")
public class MySpringBootApplication {
public static void main(String[] args) {
SpringApplication.run(MySpringBootApplication.class, args);
}
}
```
相关推荐
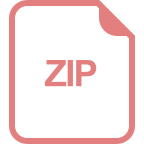
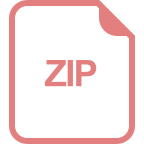
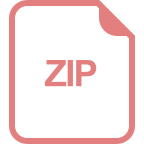



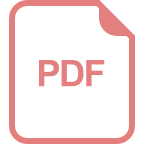
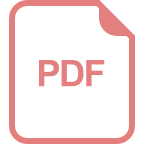
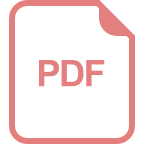
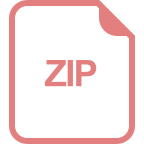
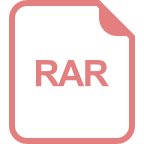