cruds() { return CRUD({ title: '公告管理', url: 'api/notice', idField: 'id', sort: 'id,desc', crudMethod: { ...crudNotice }}) },能从后端@RequestMapping("/api/notice")中获取数据吗?怎么该写成使用fetch()方法或axios()方法?
时间: 2023-03-30 12:03:41 浏览: 310
可以从后端@RequestMapping("/api/notice")中获取数据。如果要使用fetch()方法或axios()方法,可以在前端代码中使用相应的库来发送请求,例如使用fetch()方法可以这样写:
fetch('/api/notice')
.then(response => response.json())
.then(data => console.log(data))
使用axios()方法可以这样写:
axios.get('/api/notice')
.then(response => console.log(response.data))
.catch(error => console.log(error))
相关问题
这段代码中那段是负责从@RequestMapping("/api/notice")中获取数据的?如何将获取数据的那段代码改写成fetch()或axios()方法适配该段代码进行改写获取数据?<script> import crudNotice from '@/api/notice' import CRUD, { presenter, header, form, crud } from '@crud/crud' import rrOperation from '@crud/RR.operation' import crudOperation from '@crud/CRUD.operation' import udOperation from '@crud/UD.operation' import pagination from '@crud/Pagination' const defaultForm = { id: null, name: null, content: null, time: null, user: null } export default { name: 'Notice', components: { pagination, crudOperation, rrOperation, udOperation }, mixins: [presenter(), header(), form(defaultForm), crud()], cruds() { return CRUD({ title: '公告管理', url: 'api/notice', idField: 'id', sort: 'id,desc', crudMethod: { ...crudNotice }}) }, data() { return { permission: { add: ['admin', 'notice:add'], edit: ['admin', 'notice:edit'], del: ['admin', 'notice:del'] }, rules: { } } }, methods: { // 钩子:在获取表格数据之前执行,false 则代表不获取数据 [CRUD.HOOK.beforeRefresh]() { return true } } } </script>
获取数据的那段代码是crudNotice,它是从@/api/notice中导入的。要将其改写成fetch()或axios()方法适配该段代码进行改写获取数据,可以这样做:
```javascript
import axios from 'axios'
const url = '/api/notice'
export default {
// 获取公告列表
getNoticeList(params) {
return axios.get(url, { params })
},
// 新增公告
addNotice(data) {
return axios.post(url, data)
},
// 修改公告
updateNotice(id, data) {
return axios.put(`${url}/${id}`, data)
},
// 删除公告
deleteNotice(id) {
return axios.delete(`${url}/${id}`)
}
}
```
然后在原来的代码中,将crudNotice改为上面的代码中的方法名即可。
vue2中的cruds
在Vue.js 2中,CRUD (Create, Read, Update, Delete) 操作是常见的数据管理操作。CRUD操作通常用于与后端API进行交互,从而实现对数据的增加、查询、更新和删除。
在Vue.js中,可以使用以下技术和概念来实现CRUD操作:
1. 组件:Vue.js的核心概念是组件化,将应用程序拆分为多个可重用的组件。可以创建各种组件来处理CRUD操作的不同方面,例如显示数据列表、创建表单、编辑表单等。
2. 数据绑定:Vue.js提供了双向数据绑定的能力,可以轻松地将数据模型与界面元素绑定在一起。这使得在用户界面上显示和修改数据变得非常方便。
3. 表单处理:Vue.js提供了表单输入绑定和事件处理的功能。可以使用v-model指令绑定表单输入字段,并在用户输入时更新数据模型。同时,可以使用事件处理器来监听表单提交事件,并将数据发送到后端API进行保存或更新。
4. HTTP请求:Vue.js本身并没有内置的HTTP请求功能,但可以使用第三方库(如axios、vue-resource等)来发送HTTP请求。通过这些库,可以与后端API进行交互,执行CRUD操作。
下面是一个简单的示例,演示了如何在Vue.js 2中执行CRUD操作:
```vue
<template>
<div>
<ul>
<li v-for="item in items" :key="item.id">
{{ item.name }}
<button @click="editItem(item)">编辑</button>
<button @click="deleteItem(item)">删除</button>
</li>
</ul>
<form @submit.prevent="addItem">
<input type="text" v-model="newItemName" placeholder="请输入项目名称" />
<button type="submit">添加</button>
</form>
</div>
</template>
<script>
export default {
data() {
return {
items: [], // 存储项目列表
newItemName: '' // 新项目的名称
};
},
methods: {
// 获取项目列表
fetchItems() {
// 发送HTTP请求,获取项目列表数据
// axios.get('/api/items')
// .then(response => {
// this.items = response.data;
// })
// .catch(error => {
// console.error(error);
// });
},
// 添加项目
addItem() {
// 发送HTTP请求,将新项目保存到后端API
// axios.post('/api/items', { name: this.newItemName })
// .then(response => {
// this.fetchItems(); // 刷新项目列表
// this.newItemName = ''; // 清空输入框
// })
// .catch(error => {
// console.error(error);
// });
},
// 编辑项目
editItem(item) {
// 打开编辑表单,允许用户修改项目名称等信息
},
// 删除项目
deleteItem(item) {
// 发送HTTP请求,从后端API删除指定项目
// axios.delete(`/api/items/${item.id}`)
// .then(response => {
// this.fetchItems(); // 刷新项目列表
// })
// .catch(error => {
// console.error(error);
// });
}
},
mounted() {
this.fetchItems(); // 在组件加载后获取项目列表
}
};
</script>
```
这只是一个简单的示例,演示了如何使用Vue.js 2来处理CRUD操作。具体的实现方式可能会因你的应用程序需求而有所不同。
相关推荐
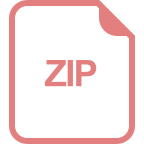
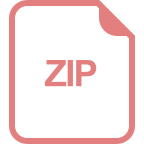
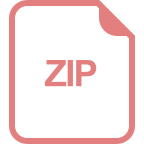
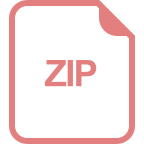
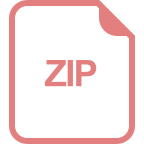
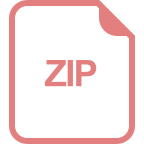
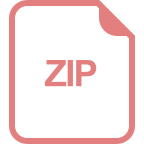
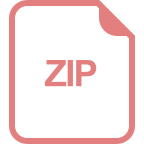
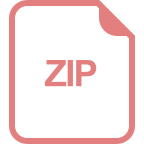
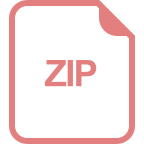
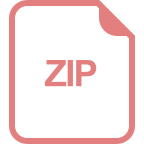
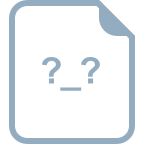
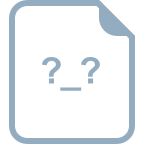