html表单数据传给后端
时间: 2023-09-05 21:10:38 浏览: 58
要将 HTML 表单数据传递给后端,需要使用一种 Web 技术,比如 AJAX 或者表单提交。
使用 AJAX:
1. 在 JavaScript 中获取表单数据。
2. 创建一个 XMLHttpRequest 对象。
3. 使用 XMLHttpRequest 对象向后端发送数据。
4. 使用回调函数处理响应。
示例代码:
```
// 获取表单数据
var form = document.getElementById('myForm');
var formData = new FormData(form);
// 创建 XMLHttpRequest 对象
var xhr = new XMLHttpRequest();
// 发送数据
xhr.open('POST', '/backend/api', true);
xhr.send(formData);
// 处理响应
xhr.onreadystatechange = function() {
if (xhr.readyState === 4 && xhr.status === 200) {
console.log(xhr.responseText);
}
};
```
使用表单提交:
1. 在 HTML 中创建一个表单元素,并设置 action 和 method 属性。
2. 在表单中添加需要提交的表单元素。
3. 在后端接收表单数据。
示例代码:
```
<form action="/backend/api" method="POST">
<input type="text" name="username">
<input type="password" name="password">
<button type="submit">提交</button>
</form>
```
在后端接收表单数据:
```
from flask import Flask, request
app = Flask(__name__)
@app.route('/backend/api', methods=['POST'])
def api():
username = request.form['username']
password = request.form['password']
# 处理表单数据
return 'success'
```
相关推荐
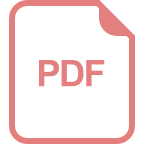
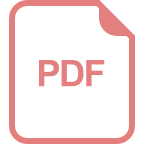
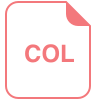













