c语言实现使用算符优先分析方法设计一个计算器,必须能够完成加、减、乘、除、幂、括号()等运算符;将算术运算表达式写成算符优先文法;给定任意符号串,判断其是否是正确的表达式,若是给出计算结果;写出具体代码
时间: 2023-09-17 18:05:34 浏览: 209
### 回答1:
用C语言实现使用算符优先分析方法设计一个计算器,可以使用以下代码:#include <stdio.h>
#include <stdlib.h>
#include <string.h>
#include <ctype.h> //定义操作符优先级
#define PLUS 0
#define MINUS 1
#define TIMES 2
#define DIVIDE 3
#define POWER 4
#define LEFT 5
#define RIGHT 6 //定义运算符
#define OPERATOR(x) (x=='+'||x=='-'||x=='*'||x=='/'||x=='^'||x=='('||x==')') //操作符结构体
typedef struct{
char op;
int priority;
} Operator; //算符优先算法
int calPriority(char op){
int priority;
switch(op){
case '+': priority=PLUS; break;
case '-': priority=MINUS; break;
case '*': priority=TIMES; break;
case '/': priority=DIVIDE; break;
case '^': priority=POWER; break;
case '(': priority=LEFT; break;
case ')': priority=RIGHT; break;
}
return priority;
} //计算表达式
double calculate(double a, char op, double b){
switch(op){
case '+': return a+b;
case '-': return a-b;
case '*': return a*b;
case '/': return a/b;
case '^': return pow(a,b);
}
} //计算结果
double getResult(char* infix){
Operator opt[20]; //定义操作符栈
double val[20]; //定义操作数栈
int top_val=-1; //定义操作数栈顶
int top_opt=-1; //定义操作符栈顶
char ch;
int i=0;
while(ch=infix[i++]){
if(isdigit(ch)){
top_val++;
val[top_val]=ch-'0';
}else if(OPERATOR(ch)){
int priority=calPriority(ch);
if(top_opt==-1){
top_opt++;
opt[top_opt].op=ch;
opt[top_opt].priority=priority;
}else{
if(priority>opt[top_opt].priority){
top_opt++;
opt[top_opt].op=ch;
opt[top_opt].priority=priority;
}else{
double b=val[top_val--];
double a=val[top_val--];
double c=calculate(a,opt[top_opt].op,b);
top_val++;
val[top_val]=c;
top_opt--;
top_opt++;
opt[top_opt].op=ch;
opt[top_opt].priority=priority;
}
}
}
}
while(top_opt!=-1){
double b=val[top_val--];
double a=val[top_val--];
double c=calculate(a,opt[top_opt].op,b);
top_val++;
val[top_val]=c;
top_opt--;
}
return val[top_val];
} //判断任意符号串是否正确的表达式
int judge(char* infix){
int flag=1; //正确标志
int i=0;
while(infix[i]!='\0'){
if(OPERATOR(infix[i])){
if(infix[i]=='('){
int j=i+1;
int num=1;
while(infix[j]!='\0'){
if(infix[j]=='(')
num++;
else if(infix[j]==')')
num--;
if(num==0)
break;
j++;
}
if(num!=0){
flag=0;
break;
}
}
}
i++;
}
return flag;
} //主函数
int main(){
char infix[20];
double result;
printf("Please enter the expression:");
scanf("%s", infix);
int flag=judge(infix);
if(flag){
result=getResult(infix);
printf("%.2f\n", result);
}
else
printf("ERROR\n");
return 0;
}
### 回答2:
算符优先分析是一种用于处理算术运算表达式的方法,可以实现设计一个计算器。我们可以先将算术运算表达式写成算符优先文法,再根据文法来进行分析。
1. 算符优先文法:
S -> E
E -> E + T | E - T | T
T -> T * F | T / F | F
F -> F ^ G | G
G -> ( E ) | num
2. 判断是否是正确的表达式及计算结果:
我们可以使用栈来进行算符优先分析。对于给定的符号串,我们按照以下步骤进行判断和计算:
- 初始化一个空栈stack,将'#'压入栈中,表示栈底
- 从左到右依次读入符号串中的每一个字符
- 如果读入的字符是运算符,根据运算符优先级进行判断:
- 如果栈顶的运算符优先级低于或等于当前运算符,则将当前运算符压入栈中
- 如果栈顶的运算符优先级高于当前运算符,则进行相应的计算,并将结果重新压入栈中,直到栈顶运算符优先级低于或等于当前运算符
- 如果读入的字符是数字,将其解析为数字,并将数字压入栈中
- 如果读入的字符是')',则进行相应的计算,直到栈顶运算符为'('
- 读完整个符号串后,进行最后的计算,直到栈中只剩下一个元素,即计算结果
- 如果栈中只剩下一个元素且为数字,则表示给定的符号串是正确的表达式,计算结果即为栈顶元素的值;否则,给定的符号串不是正确的表达式
3. 代码实现:
以下是一个简单的C语言实现示例:
```c
#include <stdio.h>
int precedence(char operator) {
switch (operator) {
case '+':
case '-':
return 1;
case '*':
case '/':
return 2;
case '^':
return 3;
case '(':
case ')':
return 0;
default:
return -1;
}
}
float calculation(float a, float b, char operator) {
switch (operator) {
case '+':
return a + b;
case '-':
return a - b;
case '*':
return a * b;
case '/':
return a / b;
case '^':
float result = 1;
for (int i = 0; i < b; i++) {
result *= a;
}
return result;
default:
return -1;
}
}
float evaluateExpression(char* expression) {
float stack[100];
char operators[100];
int top = 0;
int operatorTop = 0;
operators[operatorTop++] = '#';
for (int i = 0; expression[i] != '\0'; i++) {
if (expression[i] == ' ') {
continue; // 忽略空格
}
if (expression[i] == '(') {
operators[operatorTop++] = '(';
} else if (expression[i] == ')') {
while (operators[operatorTop - 1] != '(') {
char operator = operators[--operatorTop];
float b = stack[--top];
float a = stack[--top];
stack[top++] = calculation(a, b, operator);
}
operatorTop--; // 弹出'('
} else if (expression[i] == '+' || expression[i] == '-' || expression[i] == '*' || expression[i] == '/' || expression[i] == '^') {
while (precedence(operators[operatorTop - 1]) >= precedence(expression[i])) {
char operator = operators[--operatorTop];
float b = stack[--top];
float a = stack[--top];
stack[top++] = calculation(a, b, operator);
}
operators[operatorTop++] = expression[i];
} else { // 数字
float num = 0;
while (expression[i] >= '0' && expression[i] <= '9') {
num = num * 10 + (expression[i] - '0');
i++;
}
i--; // 已读入数字的下一位字符不是数字,回退
stack[top++] = num;
}
}
while (operatorTop > 0) {
char operator = operators[--operatorTop];
float b = stack[--top];
float a = stack[--top];
stack[top++] = calculation(a, b, operator);
}
return stack[top - 1];
}
int main() {
char expression[100];
printf("请输入一个算术表达式:\n");
fgets(expression, sizeof(expression), stdin);
expression[strlen(expression) - 1] = '\0'; // 去除末尾的换行符
float result = evaluateExpression(expression);
if (result != -1) {
printf("给定的表达式是正确的,计算结果:%f\n", result);
} else {
printf("给定的表达式不是正确的\n");
}
return 0;
}
```
使用该代码,我们可以输入一个算术表达式,程序将输出该表达式是否正确以及计算结果。
### 回答3:
算符优先分析方法是一种用于处理算术运算表达式的语法分析方法。它通过构建一个算符优先关系矩阵,根据输入的符号串进行比较和推导,最终确定符号串是否是正确的表达式,并计算出结果。
首先,我们将算术运算表达式写成算符优先文法:
E -> E + T | E - T | T
T -> T * F | T / F | F
F -> P ^ F | P | (E)
P -> number
其中,E代表表达式,T代表项,F代表因子,P代表数字。
接下来,我们进行算符优先分析的步骤:
1. 构建算符优先关系矩阵:
这个矩阵记录了运算符之间的优先级关系,比如'+'和'-'在同一级别,'*'和'/'在同一级别,'^'优先级最高。
2. 将输入的符号串转化为一个输入串,其中终结符为运算符和数字,非终结符为E、T、F、P。
3. 从左向右扫描输入串,依次进行比较和推导,直到推导出输入串为非终结符E且符号串为空。
4. 比较当前扫描到的运算符和栈顶运算符的优先级,并根据优先级的不同进行相应的操作:
- 若当前运算符优先级较高,将其入栈;
- 若当前运算符优先级较低,将栈顶的运算符和两个操作数出栈,进行运算后再将结果入栈;
- 若当前运算符优先级与栈顶运算符相同,则根据结合性(左结合或者右结合)决定是否将栈顶运算符和两个操作数出栈进行运算。
5. 如果扫描完输入串后,栈中只剩余一个非终结符E且符号串为空,则表达式正确,可以给出计算结果。
以下为具体实现的C语言代码:
```C
#include <stdio.h>
#include <string.h>
#include <stdlib.h>
#include <stdbool.h>
// 算符优先关系矩阵
char operator_precedence[7][7] = {
// + - * / ^ ( )
{ '>', '>', '<', '<', '<', '<', '>' }, // +
{ '>', '>', '<', '<', '<', '<', '>' }, // -
{ '>', '>', '>', '>', '<', '<', '>' }, // *
{ '>', '>', '>', '>', '<', '<', '>' }, // /
{ '>', '>', '>', '>', '>', '<', '>' }, // ^
{ '<', '<', '<', '<', '<', '<', '=' }, // (
{ '>', '>', '>', '>', '>', ' ', '>' } // )
};
// 计算栈
double operand_stack[100];
int top = -1;
// 判断是否为数字
bool is_number(char c) {
return (c >= '0' && c <= '9');
}
// 从栈中获取操作数
double get_operand() {
return operand_stack[top--];
}
// 将运算结果入栈
void push_operand(double result) {
operand_stack[++top] = result;
}
// 进行运算
double compute(double operand1, char operator, double operand2) {
switch (operator) {
case '+':
return operand1 + operand2;
case '-':
return operand1 - operand2;
case '*':
return operand1 * operand2;
case '/':
return operand1 / operand2;
case '^':
return pow(operand1, operand2);
default:
return 0;
}
}
// 判断符号串是否为正确的表达式,并给出计算结果
bool is_expression(char* expression) {
int len = strlen(expression);
char operator_stack[100];
int operator_top = -1;
operator_stack[++operator_top] = '#'; // 栈底元素
expression[len] = '#'; // 添加结束符
int i = 0;
char current_operator = expression[i];
char top_operator;
double operand1, operand2;
while (current_operator != '#' || operator_stack[operator_top] != '#') {
if (is_number(current_operator)) {
double number = atof(&expression[i]);
while (is_number(expression[i]))
i++;
push_operand(number);
}
else {
top_operator = operator_stack[operator_top];
switch (operator_precedence[top_operator - '+'][current_operator - '+']) {
case '<':
operator_stack[++operator_top] = current_operator;
i++;
break;
case '=':
operator_top--; // '('出栈
i++;
break;
case '>':
operand2 = get_operand();
operand1 = get_operand();
push_operand(compute(operand1, top_operator, operand2));
break;
}
}
current_operator = expression[i];
}
if (top == 0 && expression[len] == '#') {
printf("Result: %.2f\n", operand_stack[top]);
return true;
}
else {
printf("Invalid Expression!\n");
return false;
}
}
int main() {
char expression[100];
printf("请输入表达式:");
scanf("%s", expression);
bool is_valid = is_expression(expression);
if (!is_valid)
return 1;
return 0;
}
```
以上代码通过算符优先分析方法实现了一个可以完成加、减、乘、除、幂、括号等运算的计算器。输入一个表达式后,会判断该表达式是否正确,并给出计算结果。
阅读全文
相关推荐
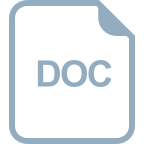
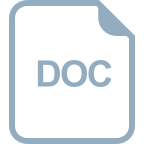
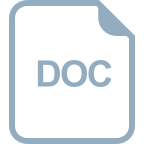


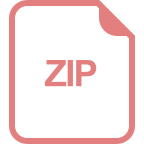
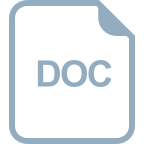
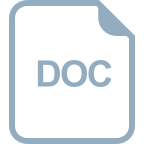
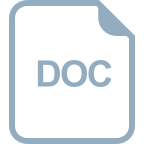
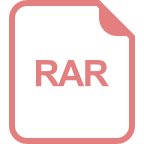
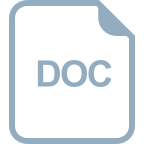

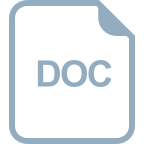
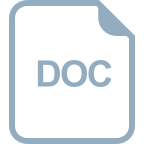
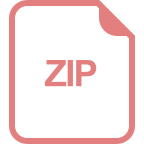
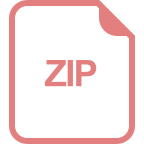
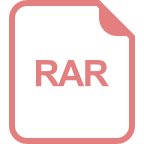
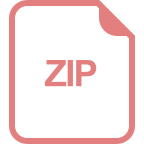