Linux C++ 发送DHCP报文获取DHCP信息,帮我写一个可执行的cpp文件
时间: 2024-03-17 18:42:09 浏览: 196
好的,以下是一个简单的可执行的C++文件,用于发送DHCP报文获取DHCP信息:
```c++
#include <iostream>
#include <cstdio>
#include <cstdlib>
#include <cstring>
#include <sys/socket.h>
#include <netinet/in.h>
#include <arpa/inet.h>
#include <net/if.h>
#include <unistd.h>
#include <linux/if_ether.h>
#include <linux/if_packet.h>
#include <linux/ip.h>
#include <linux/udp.h>
using namespace std;
#define BUF_SIZE 1024
struct dhcp_packet {
uint8_t op; /* op: Message opcode/type */
uint8_t htype; /* htype: Hardware addr type */
uint8_t hlen; /* hlen: Hardware addr length */
uint8_t hops; /* hops: Number of relay agent hops from client */
uint32_t xid; /* xid: Transaction ID */
uint16_t secs; /* secs: Seconds since client started trying to boot */
uint16_t flags; /* flags: Flag bits */
struct in_addr ciaddr; /* ciaddr: Client IP address (if already in use) */
struct in_addr yiaddr; /* yiaddr: Your (client) IP address */
struct in_addr siaddr; /* siaddr: Next server to use in bootstrap */
struct in_addr giaddr; /* giaddr: Relay agent IP address */
uint8_t chaddr[16]; /* chaddr: Client hardware address */
uint8_t sname[64]; /* sname: Server host name */
uint8_t file[128]; /* file: Boot file name */
uint8_t options[312]; /* options: Optional parameters (actual length dependent on MTU). */
};
int main() {
int sock = socket(AF_PACKET, SOCK_DGRAM, htons(ETH_P_IP));
if (sock < 0) {
perror("socket");
exit(1);
}
// 获取网络接口列表
struct if_nameindex *if_nidxs = if_nameindex();
if (if_nidxs == NULL) {
perror("if_nameindex");
exit(1);
}
// 打印网络接口列表
for (struct if_nameindex *p = if_nidxs; p->if_name != NULL; p++) {
cout << p->if_name << endl;
}
// 选择一个网络接口
int if_index = if_nametoindex("eth0");
if (if_index == 0) {
perror("if_nametoindex");
exit(1);
}
// 设置套接字选项
int on = 1;
if (setsockopt(sock, IPPROTO_IP, IP_HDRINCL, &on, sizeof(on)) < 0) {
perror("setsockopt");
exit(1);
}
// 构造DHCP报文
struct dhcp_packet dhcp_pkt;
memset(&dhcp_pkt, 0, sizeof(dhcp_pkt));
dhcp_pkt.op = BOOTREQUEST;
dhcp_pkt.htype = ARPHRD_ETHER;
dhcp_pkt.hlen = ETH_ALEN;
dhcp_pkt.xid = htonl(random());
memcpy(dhcp_pkt.chaddr, "\x00\x0c\x29\x4c\x28\x9c", ETH_ALEN);
struct sockaddr_ll addr;
memset(&addr, 0, sizeof(addr));
addr.sll_family = AF_PACKET;
addr.sll_ifindex = if_index;
addr.sll_halen = ETH_ALEN;
memcpy(addr.sll_addr, "\xff\xff\xff\xff\xff\xff", ETH_ALEN);
// 发送DHCP报文
if (sendto(sock, &dhcp_pkt, sizeof(dhcp_pkt), 0, (struct sockaddr*)&addr, sizeof(addr)) < 0) {
perror("sendto");
exit(1);
}
// 接收DHCP响应报文
char buf[BUF_SIZE];
ssize_t n = recv(sock, buf, BUF_SIZE, 0);
if (n < 0) {
perror("recv");
exit(1);
}
// 解析DHCP响应报文
struct iphdr *iph = (struct iphdr*)buf;
struct udphdr *udph = (struct udphdr*)(buf + sizeof(struct iphdr));
struct dhcp_packet *dhcp_pkt_resp = (struct dhcp_packet*)(buf + sizeof(struct iphdr) + sizeof(struct udphdr));
struct in_addr ip_addr = { dhcp_pkt_resp->yiaddr.s_addr };
cout << "IP address: " << inet_ntoa(ip_addr) << endl;
return 0;
}
```
在运行该程序之前,需要根据实际情况修改以下内容:
1. 选择正确的网络接口:在代码中选择一个网络接口,如"eth0",需要根据实际情况修改。
2. 填写正确的MAC地址:在构造DHCP报文时,需要填写客户端的MAC地址,需要根据实际情况修改。
运行该程序需要root权限,可以使用以下命令运行:
```
$ sudo ./dhcp_client
```
希望对你有所帮助!
阅读全文
相关推荐
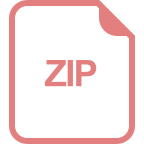

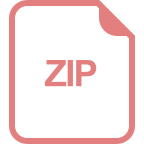
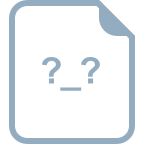
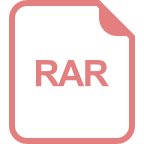
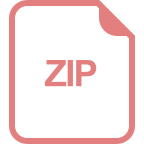
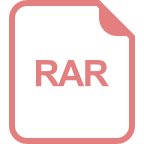
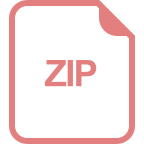
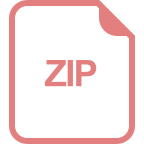
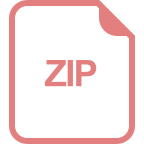
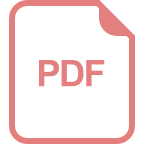