python加速for循环的方法
时间: 2023-07-05 22:27:47 浏览: 63
有多种方法可以加速 Python 的 for 循环,以下是其中几种常用的方法:
1. 使用列表推导式或生成器表达式代替 for 循环
```python
# 使用 for 循环
result = []
for i in range(10):
result.append(i * 2)
# 使用列表推导式
result = [i * 2 for i in range(10)]
# 使用生成器表达式
result = (i * 2 for i in range(10))
```
2. 使用 Numpy 的向量化操作
```python
import numpy as np
# 使用 for 循环
x = np.array([1, 2, 3, 4, 5])
y = np.array([6, 7, 8, 9, 10])
result = np.zeros_like(x)
for i in range(len(x)):
result[i] = x[i] + y[i]
# 使用向量化操作
result = x + y
```
3. 使用 Cython 或 Numba 编译代码
```python
# 使用 Cython 编译代码
%load_ext Cython
%%cython
def multiply_by_two(lst):
result = []
for i in lst:
result.append(i * 2)
return result
# 使用 Numba 编译代码
from numba import jit
@jit(nopython=True)
def multiply_by_two(lst):
result = []
for i in lst:
result.append(i * 2)
return result
```
以上是几种加速 Python for 循环的方法,不同的场景可以选择不同的方法来优化代码性能。
相关推荐
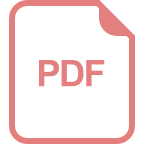
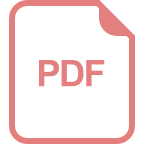
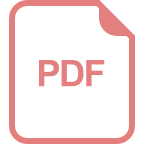













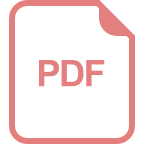
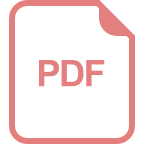