求单项链表中第i个结点的指针(函数),若不存在,则返回NULL
时间: 2023-06-08 20:04:52 浏览: 369
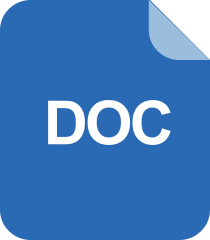
3-2 试编写一个算法,在带表头结点的单链表中寻找第i个结点.doc
以下是求单项链表中第i个结点的指针的函数实现(C语言):
```
struct ListNode* getIthNode(struct ListNode* head, int i) {
if (head == NULL || i < 1) { // 判断链表头指针和i的合法性
return NULL;
}
struct ListNode* cur = head;
while (i > 1 && cur != NULL) { // 找到第i个结点的前一个结点
cur = cur->next;
i--;
}
return cur; // 返回第i个结点的指针,如果不存在,则返回NULL
}
```
使用方法如下:
1. 定义链表结点的结构体:
```
struct ListNode {
int val;
struct ListNode *next;
};
```
2. 创建单项链表并初始化:
```
struct ListNode* head = malloc(sizeof(struct ListNode)); // 创建头结点
head->val = 1; // 初始化头结点的值
head->next = NULL; // 初始化头结点的指针
struct ListNode* cur = head; // 创建指针cur指向头结点
for (int i = 2; i <= n; i++) { // 创建n个结点,并连接成链表
struct ListNode* node = malloc(sizeof(struct ListNode));
node->val = i;
node->next = NULL;
cur->next = node;
cur = node;
}
```
3. 调用函数获取第i个结点的指针:
```
struct ListNode* ithNode = getIthNode(head, i);
if (ithNode == NULL) {
printf("第%d个结点不存在\n", i);
} else {
printf("第%d个结点的值为%d\n", i, ithNode->val);
}
```
阅读全文
相关推荐
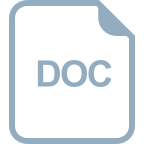
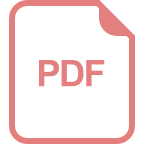

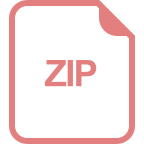
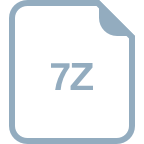
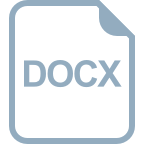
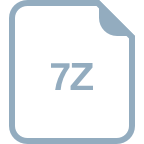
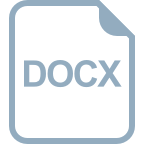