python实现卷积神经网络算法
时间: 2023-07-20 12:27:44 浏览: 104
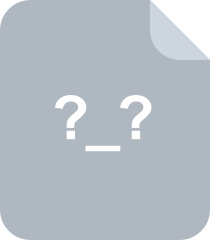
python: 基于卷积神经网络原理的实现

好的,下面是一个简单的 Python 实现卷积神经网络算法的示例:
```
import numpy as np
# 定义卷积函数
def convolution(image, kernel):
# 获取图像和卷积核的大小
image_height, image_width = image.shape
kernel_height, kernel_width = kernel.shape
# 计算卷积后的输出大小
output_height = image_height - kernel_height + 1
output_width = image_width - kernel_width + 1
# 初始化输出
output = np.zeros((output_height, output_width))
# 进行卷积操作
for i in range(output_height):
for j in range(output_width):
output[i][j] = np.sum(image[i:i+kernel_height, j:j+kernel_width] * kernel)
return output
# 定义卷积神经网络类
class ConvNet:
def __init__(self, num_filters, filter_size, stride):
self.num_filters = num_filters
self.filter_size = filter_size
self.stride = stride
# 初始化卷积核权重
self.weights = np.random.randn(num_filters, filter_size, filter_size) / (filter_size * filter_size)
# 定义前向传播函数
def forward(self, input):
# 获取输入大小
input_height, input_width = input.shape
# 计算输出大小
output_height = (input_height - self.filter_size) / self.stride + 1
output_width = (input_width - self.filter_size) / self.stride + 1
# 初始化输出
output = np.zeros((self.num_filters, output_height, output_width))
# 进行卷积操作
for i in range(self.num_filters):
for j in range(output_height):
for k in range(output_width):
output[i][j][k] = np.sum(input[j*self.stride:j*self.stride+self.filter_size, k*self.stride:k*self.stride+self.filter_size] * self.weights[i])
return output
# 测试代码
# 定义一个 5x5 的图像
image = np.array([[1, 2, 3, 4, 5],
[6, 7, 8, 9, 10],
[11, 12, 13, 14, 15],
[16, 17, 18, 19, 20],
[21, 22, 23, 24, 25]])
# 定义一个 3x3 的卷积核
kernel = np.array([[1, 0, -1],
[2, 0, -2],
[1, 0, -1]])
# 进行卷积操作
output = convolution(image, kernel)
# 输出结果
print(output)
# 创建一个卷积神经网络对象
conv_net = ConvNet(num_filters=3, filter_size=3, stride=1)
# 进行前向传播
output = conv_net.forward(image)
# 输出结果
print(output)
```
这是一个简单的实现,仅用于演示卷积神经网络算法的基本原理。在实际应用中,还需要考虑更多细节,如网络的深度、激活函数、损失函数、优化器等等。
阅读全文
相关推荐
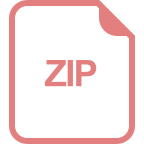
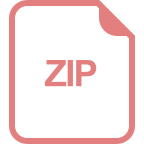
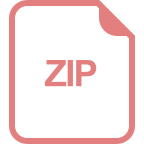
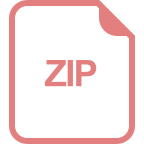
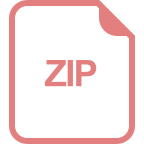
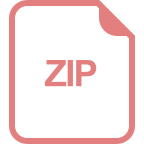
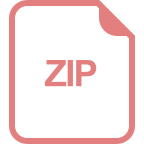
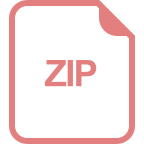
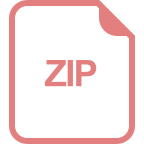
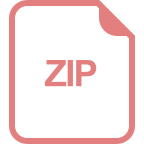
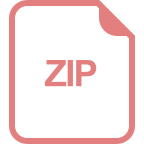
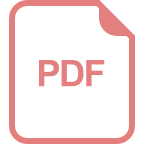
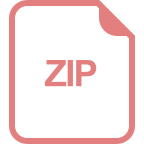
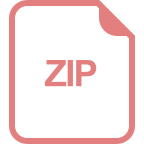
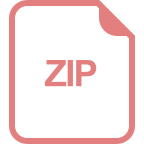
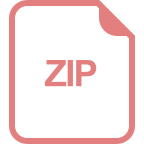
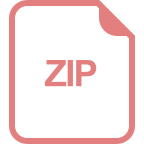