用c++代码实现一下,针对给定的二值图像,采用8链码对图像轮廓进行描述,给出链码描述结果,并计算循环首差链码。(采用C++语言实现,不用OpenCV)
时间: 2024-03-23 18:40:58 浏览: 130
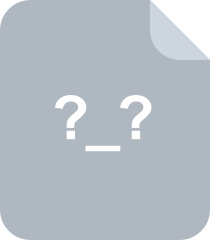
使用c语言实现图像二值化
好的,以下是用 C++ 实现的二值图像8链码和循环首差链码计算程序:
```c++
#include <iostream>
#include <vector>
#include <cmath>
using namespace std;
// 定义8个方向的偏移量
const int dx[] = {1, 1, 0, -1, -1, -1, 0, 1};
const int dy[] = {0, 1, 1, 1, 0, -1, -1, -1};
// 找到图像的起始点
pair<int, int> get_start_point(vector<vector<int>>& img) {
for (int i = 0; i < img.size(); i++) {
for (int j = 0; j < img[i].size(); j++) {
if (img[i][j] > 0) {
return make_pair(i, j);
}
}
}
return make_pair(0, 0);
}
// 计算8链码
vector<int> get_chain_code(vector<vector<int>>& img) {
auto start_point = get_start_point(img);
vector<int> chain_code;
int last_code = 0;
int x = start_point.first;
int y = start_point.second;
while (true) {
int next_x, next_y;
for (int i = 0; i < 8; i++) {
int j = (last_code + i) % 8;
next_x = x + dx[j];
next_y = y + dy[j];
if (next_x >= 0 && next_x < img.size() && next_y >= 0 && next_y < img[next_x].size() && img[next_x][next_y] > 0) {
chain_code.push_back(j);
x = next_x;
y = next_y;
last_code = j;
break;
}
}
if (make_pair(x, y) == start_point && last_code == chain_code[0]) {
break;
}
}
return chain_code;
}
// 计算循环首差链码
vector<int> get_circular_shifted_chain_code(vector<int>& chain_code) {
vector<int> circular_shifted_chain_code;
for (int i = 0; i < chain_code.size(); i++) {
circular_shifted_chain_code.push_back((chain_code[i] - chain_code[0] + 8) % 8);
}
return circular_shifted_chain_code;
}
int main() {
// 读取二值图像,假设已经将其存储在一个二维数组 img 中
vector<vector<int>> img = {{0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 1, 1, 1, 0, 0},
{0, 0, 0, 1, 0, 1, 0, 0},
{0, 0, 0, 1, 1, 1, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0},
{0, 0, 0, 0, 0, 0, 0, 0}};
// 计算8链码
vector<int> chain_code = get_chain_code(img);
cout << "8链码:";
for (int i = 0; i < chain_code.size(); i++) {
cout << chain_code[i] << " ";
}
cout << endl;
// 计算循环首差链码
vector<int> circular_shifted_chain_code = get_circular_shifted_chain_code(chain_code);
cout << "循环首差链码:";
for (int i = 0; i < circular_shifted_chain_code.size(); i++) {
cout << circular_shifted_chain_code[i] << " ";
}
cout << endl;
return 0;
}
```
其中,二值图像被存储在一个二维数组 `img` 中,每个元素为 0 或 1。程序中假设图像大小为 8x8,其中轮廓部分用 1 表示,背景用 0 表示。你可以将 `img` 数组替换为你自己的二值图像数组。
阅读全文
相关推荐


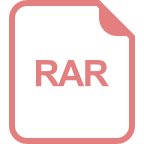
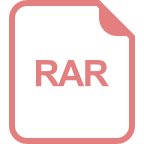
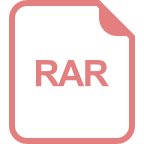
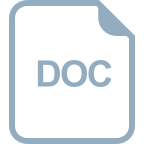
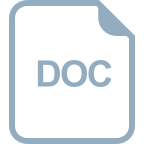
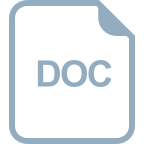
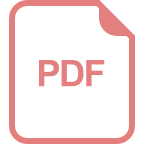
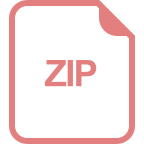
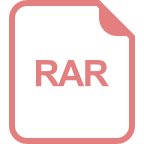
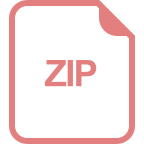