复杂json解析多条数据分别插入orace不同的表java代码
时间: 2024-03-12 16:45:33 浏览: 120
假设你有一个 JSON 数据,其中包含多个对象,每个对象表示一个人的信息。现在你需要将每个人的信息插入到不同的 Oracle 数据库表中。以下是一个可能的 Java 代码示例:
```java
import java.sql.Connection;
import java.sql.DriverManager;
import java.sql.PreparedStatement;
import java.sql.SQLException;
import org.json.JSONArray;
import org.json.JSONObject;
public class JsonToOracle {
public static void main(String[] args) {
String jsonStr = "your JSON string"; // 假设你已经拿到了 JSON 字符串
JSONArray jsonArray = new JSONArray(jsonStr); // 将 JSON 字符串转换为 JSONArray
try (Connection conn = DriverManager.getConnection("your Oracle connection string")) {
// 获取数据库连接
// 针对每个人的信息,分别插入到不同的表中
for (int i = 0; i < jsonArray.length(); i++) {
JSONObject jsonObject = jsonArray.getJSONObject(i);
// 从 JSONObject 中获取需要插入到表中的数据
String name = jsonObject.getString("name");
int age = jsonObject.getInt("age");
String address = jsonObject.getString("address");
// 根据需要插入到的表名,构造 SQL 语句
String tableName = "your table name";
String sql = "INSERT INTO " + tableName + " (name, age, address) VALUES (?, ?, ?)";
// 创建 PreparedStatement 对象,并填充占位符
try (PreparedStatement pstmt = conn.prepareStatement(sql)) {
pstmt.setString(1, name);
pstmt.setInt(2, age);
pstmt.setString(3, address);
pstmt.executeUpdate(); // 执行 SQL,将数据插入到表中
}
}
} catch (SQLException e) {
e.printStackTrace();
}
}
}
```
需要注意的是,上面的代码仅是一个示例,实际情况可能更加复杂,例如需要对插入的数据进行校验、处理重复数据等。因此,实际开发中需要根据具体需求进行调整。
阅读全文
相关推荐
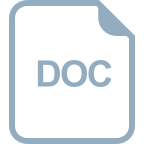
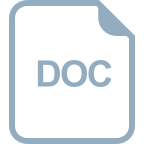
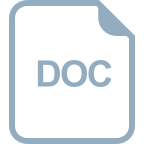

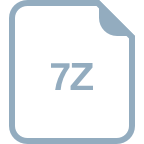
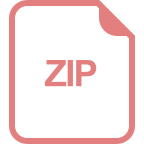
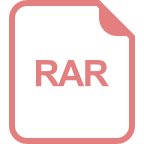
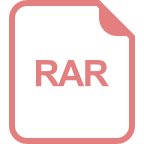
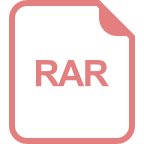
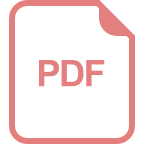
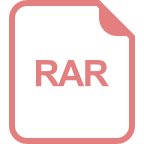
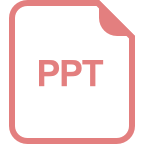
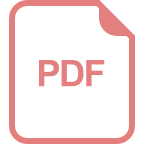
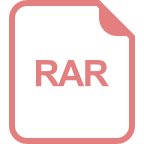
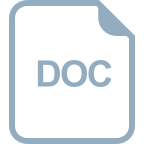


