import pyinotify class EventHandler(pyinotify.ProcessEvent): def __init__(self, path): self.path = path def process_IN_MODIFY(self, event): print("File modified:", event.pathname) wm = pyinotify.WatchManager() notifier = pyinotify.Notifier(wm) paths = ['/opt/tomcat/logs', '/home/temp'] for path in paths: wm.add_watch(path, pyinotify.IN_MODIFY, rec=True, auto_add=True, event_class=EventHandler(path)) notifier.loop()代码报错:TypeError: add_watch() got an unexpected keyword argument 'event_class'
时间: 2024-01-11 16:03:14 浏览: 13
这个错误是因为在Pyinotify 0.9.6 版本中已经删除了`event_class`参数,所以需要升级到最新版本的Pyinotify。或者,你可以使用以下代码替换最后一行:
```
notifier.loop(event_loop=pyinotify.EventLoop(event_class=EventHandler(path)))
```
这里使用了`event_loop`参数,并将`event_class`作为参数传递给`EventHandler`。这样可以实现相同的效果。
相关问题
import pyinotify class EventHandler(pyinotify.ProcessEvent): def __init__(self, path): self.path = path def process_IN_MODIFY(self, event): print("File modified:", event.pathname) wm = pyinotify.WatchManager() notifier = pyinotify.Notifier(wm, EventHandler()) paths = ['/opt/tomcat/logs', '/home/temp'] for path in paths: wm.add_watch(path, pyinotify.IN_MODIFY, rec=True, auto_add=True) notifier.loop()报错: notifier = pyinotify.Notifier(wm, EventHandler()) TypeError: __init__() takes exactly 2 arguments (1 given)
这个错误提示说明 `EventHandler` 类的 `__init__` 方法需要传入 `path` 参数,但是在 `pyinotify.Notifier` 实例化时没有传入该参数,因此需要在实例化 `EventHandler` 时传入 `path` 参数,例如:
```python
import pyinotify
class EventHandler(pyinotify.ProcessEvent):
def __init__(self, path):
self.path = path
def process_IN_MODIFY(self, event):
print("File modified:", event.pathname)
wm = pyinotify.WatchManager()
notifier = pyinotify.Notifier(wm, EventHandler('/opt/tomcat/logs'))
wm.add_watch('/opt/tomcat/logs', pyinotify.IN_MODIFY, rec=True, auto_add=True)
notifier.loop()
```
注意,`wm.add_watch` 也需要改为仅传入一个路径参数。如果你想监控多个路径,可以在循环中分别创建 `EventHandler` 实例,并为每个实例传入相应的路径参数。
如何利用 pyinotify 在一个python脚本中,监控多个路径
可以使用pyinotify中的WatchManager来监控多个路径,具体步骤如下:
1. 创建WatchManager对象并实例化一个Notifier对象。
```
import pyinotify
wm = pyinotify.WatchManager()
notifier = pyinotify.Notifier(wm)
```
2. 为每个要监控的路径创建一个ProcessEvent子类,并在类的process_IN_MODIFY方法中定义响应逻辑。
```
class EventHandler(pyinotify.ProcessEvent):
def __init__(self, path):
self.path = path
def process_IN_MODIFY(self, event):
print("File modified:", event.pathname)
```
3. 将每个路径和其对应的ProcessEvent子类实例添加到WatchManager中。
```
paths = ['/path/to/dir1', '/path/to/dir2', '/path/to/dir3']
for path in paths:
wm.add_watch(path, pyinotify.IN_MODIFY, rec=True, auto_add=True, event_class=EventHandler)
```
其中,add_watch方法的参数说明如下:
- path:要监控的路径。
- pyinotify.IN_MODIFY:要监控的事件类型(这里是文件修改事件)。
- rec=True:递归地监控子目录。
- auto_add=True:自动添加新创建的子目录。
- event_class=EventHandler:要使用的ProcessEvent子类。
4. 启动Notifier对象,开始监控。
```
notifier.loop()
```
完整代码示例:
```
import pyinotify
class EventHandler(pyinotify.ProcessEvent):
def __init__(self, path):
self.path = path
def process_IN_MODIFY(self, event):
print("File modified:", event.pathname)
wm = pyinotify.WatchManager()
notifier = pyinotify.Notifier(wm)
paths = ['/path/to/dir1', '/path/to/dir2', '/path/to/dir3']
for path in paths:
wm.add_watch(path, pyinotify.IN_MODIFY, rec=True, auto_add=True, event_class=EventHandler(path))
notifier.loop()
```
相关推荐
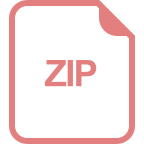
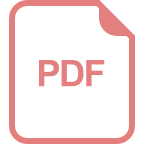




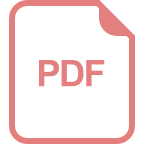
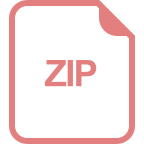
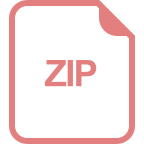
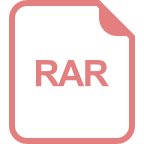
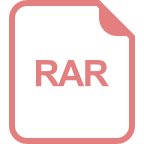