c++编写一个单词统计程序
时间: 2024-03-14 19:43:12 浏览: 78
好的,下面是一个简单的 C++ 单词统计程序:
```c++
#include <iostream>
#include <string>
#include <map>
#include <sstream>
using namespace std;
int main() {
// 初始化计数器
map<string, int> wordCount;
// 读入输入文本
stringstream ss;
string line;
while (getline(cin, line)) {
ss << line << " ";
}
// 分割单词并统计
string word;
while (ss >> word) {
++wordCount[word];
}
// 输出结果
for (const auto& pair : wordCount) {
cout << pair.first << ": " << pair.second << endl;
}
return 0;
}
```
该程序首先读入一段文本,然后将其分割成单词,并统计每个单词出现的次数,最后输出结果。其中使用了 `map` 来记录每个单词的计数器。
相关问题
c++编写一个单词统计程序: 1)打开四个文本文档,读取数据; 2)统计英文单词的数量。
好的,下面是一个 C++ 单词统计程序,可以打开四个文本文档,读取数据,统计英文单词的数量:
```c++
#include <iostream>
#include <string>
#include <fstream>
#include <sstream>
#include <map>
using namespace std;
// 统计单词数量的函数
void countWords(map<string, int>& wordCount, string& line) {
string word;
stringstream ss(line);
while (ss >> word) {
// 剔除单词中的标点符号
while (!isalpha(word.back())) {
word.pop_back();
}
// 统计单词
++wordCount[word];
}
}
int main() {
// 打开四个文本文档
ifstream file1("text1.txt");
ifstream file2("text2.txt");
ifstream file3("text3.txt");
ifstream file4("text4.txt");
// 初始化计数器
map<string, int> wordCount;
// 读取文本并统计单词
string line;
while (getline(file1, line)) {
countWords(wordCount, line);
}
while (getline(file2, line)) {
countWords(wordCount, line);
}
while (getline(file3, line)) {
countWords(wordCount, line);
}
while (getline(file4, line)) {
countWords(wordCount, line);
}
// 输出结果
cout << "英文单词数量:" << wordCount.size() << endl;
return 0;
}
```
该程序通过 `ifstream` 打开四个文本文档,然后逐行读取文本并统计单词数量。其中 `countWords` 函数用于分割单词,并统计每个单词出现的次数。最后,程序输出统计结果,即英文单词的数量。注意,为了准确地统计单词数量,程序在分割单词时剔除了单词中的标点符号。
用c++编写一个程序读取一个字符串并统计其中每个单词的长度然后输出每个单词及其对应的长度。
在C++中,你可以使用`cin`从用户那里获取输入,`istringstream`将输入分割成单词,然后遍历这些单词计算它们的长度。下面是一个简单的示例程序:
```cpp
#include <iostream>
#include <sstream>
#include <string>
int main() {
std::string input;
std::cout << "请输入一个字符串: ";
getline(std::cin, input); // 使用getline读入一行,包括空格
std::istringstream iss(input);
std::string word;
while (iss >> word) { // 逐词分割
int length = word.length();
std::cout << "单词: " << word << ", 长度: " << length << std::endl;
}
return 0;
}
```
在这个程序中,我们首先提示用户输入一个字符串,然后通过`getline`函数一次读入一行文本,而不是遇到空格就停止。接下来,我们将这个输入流传递给`istringstream`,它会按空格分隔字符串。对于每一行中的每一个单词,我们都会计算其长度,并输出。
阅读全文
相关推荐
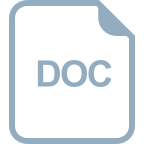
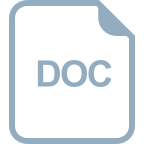











