基于canvas在浏览器图像上画线 然后让线条自动吸附到人脑医学影像图片灰色或白色区域
时间: 2023-05-28 12:04:24 浏览: 56
这个任务需要将医学影像图片加载到canvas中,并使用canvas提供的API在图片上绘制线条。然后需要检测线条与灰色或白色区域的碰撞,即线条是否落在这些区域内,如果没有,则需要将线条自动吸附到最近的灰色或白色区域边缘。
具体实现步骤如下:
1. 加载医学影像图片到canvas中,可以使用canvas提供的drawImage方法。
```javascript
const img = new Image();
img.src = 'path/to/image';
img.onload = () => {
ctx.drawImage(img, 0, 0);
};
```
2. 绘制线条,可以使用canvas提供的path和stroke方法。
```javascript
ctx.beginPath();
ctx.moveTo(x1, y1);
ctx.lineTo(x2, y2);
ctx.stroke();
```
3. 检测线条与灰色或白色区域的碰撞,可以使用canvas提供的getImageData方法获取图片像素数据,并判断像素颜色是否为灰色或白色。
```javascript
const imageData = ctx.getImageData(x, y, width, height);
const pixelData = imageData.data;
const pixelIndex = (y * width + x) * 4;
const isGray = pixelData[pixelIndex] === pixelData[pixelIndex + 1] && pixelData[pixelIndex + 1] === pixelData[pixelIndex + 2];
const isWhite = pixelData[pixelIndex] === 255 && pixelData[pixelIndex + 1] === 255 && pixelData[pixelIndex + 2] === 255;
```
4. 将线条自动吸附到最近的灰色或白色区域边缘,可以计算线条与每个灰色或白色像素点的距离,并选择距离最近的点作为吸附点。
```javascript
const grayPoints = [];
const whitePoints = [];
// 找出所有灰色和白色像素点的位置
for (let y = 0; y < height; y++) {
for (let x = 0; x < width; x++) {
const index = (y * width + x) * 4;
const isGray = pixelData[index] === pixelData[index + 1] && pixelData[index + 1] === pixelData[index + 2];
const isWhite = pixelData[index] === 255 && pixelData[index + 1] === 255 && pixelData[index + 2] === 255;
if (isGray) {
grayPoints.push({ x, y });
} else if (isWhite) {
whitePoints.push({ x, y });
}
}
}
// 计算线条与每个灰色和白色像素点的距离,并选择距离最近的点作为吸附点
let minDistance = Infinity;
let nearestPoint = null;
const points = isGray ? grayPoints : whitePoints;
points.forEach((point) => {
const distance = Math.sqrt((point.x - x) ** 2 + (point.y - y) ** 2);
if (distance < minDistance) {
minDistance = distance;
nearestPoint = point;
}
});
if (nearestPoint) {
// 将线条的终点移动到吸附点位置
x2 = nearestPoint.x;
y2 = nearestPoint.y;
}
```
完整的代码示例:
```html
<canvas id="canvas" width="800" height="600"></canvas>
<script>
const canvas = document.getElementById('canvas');
const ctx = canvas.getContext('2d');
const img = new Image();
img.src = 'path/to/image';
img.onload = () => {
ctx.drawImage(img, 0, 0);
};
let x1, y1, x2, y2;
let isDrawing = false;
canvas.addEventListener('mousedown', (event) => {
x1 = event.offsetX;
y1 = event.offsetY;
isDrawing = true;
});
canvas.addEventListener('mousemove', (event) => {
if (!isDrawing) return;
x2 = event.offsetX;
y2 = event.offsetY;
// 绘制线条
ctx.beginPath();
ctx.moveTo(x1, y1);
ctx.lineTo(x2, y2);
ctx.stroke();
// 检测线条与灰色或白色区域的碰撞,并将线条吸附到边缘
const imageData = ctx.getImageData(x2, y2, 1, 1);
const pixelData = imageData.data;
const isGray = pixelData[0] === pixelData[1] && pixelData[1] === pixelData[2];
const isWhite = pixelData[0] === 255 && pixelData[1] === 255 && pixelData[2] === 255;
if (isGray || isWhite) {
const width = canvas.width;
const height = canvas.height;
const grayPoints = [];
const whitePoints = [];
// 找出所有灰色和白色像素点的位置
for (let y = 0; y < height; y++) {
for (let x = 0; x < width; x++) {
const index = (y * width + x) * 4;
const isGray = pixelData[index] === pixelData[index + 1] && pixelData[index + 1] === pixelData[index + 2];
const isWhite = pixelData[index] === 255 && pixelData[index + 1] === 255 && pixelData[index + 2] === 255;
if (isGray) {
grayPoints.push({ x, y });
} else if (isWhite) {
whitePoints.push({ x, y });
}
}
}
// 计算线条与每个灰色和白色像素点的距离,并选择距离最近的点作为吸附点
let minDistance = Infinity;
let nearestPoint = null;
const points = isGray ? grayPoints : whitePoints;
points.forEach((point) => {
const distance = Math.sqrt((point.x - x2) ** 2 + (point.y - y2) ** 2);
if (distance < minDistance) {
minDistance = distance;
nearestPoint = point;
}
});
if (nearestPoint) {
// 将线条的终点移动到吸附点位置
x2 = nearestPoint.x;
y2 = nearestPoint.y;
}
}
});
canvas.addEventListener('mouseup', () => {
isDrawing = false;
});
</script>
```
相关推荐
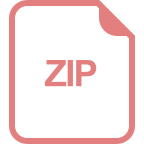
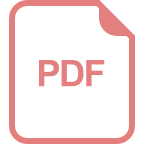














