jsch 密钥登录下载sftp文件 代码
时间: 2024-05-07 12:21:08 浏览: 12
可以使用 JSch 库实现 SFTP 文件下载,以下是一个示例代码:
```java
import com.jcraft.jsch.*;
import java.io.*;
public class SFTPDownloader {
public static void downloadFile(String host, int port, String username, String privateKeyPath, String remoteFilePath, String localFilePath)
throws JSchException, IOException {
JSch jsch = new JSch();
jsch.addIdentity(privateKeyPath);
Session session = jsch.getSession(username, host, port);
session.setConfig("StrictHostKeyChecking", "no");
session.connect();
ChannelSftp sftpChannel = (ChannelSftp) session.openChannel("sftp");
sftpChannel.connect();
InputStream inputStream = null;
try {
inputStream = sftpChannel.get(remoteFilePath);
} catch (SftpException e) {
throw new RuntimeException("Failed to download remote file: " + remoteFilePath, e);
}
OutputStream outputStream = null;
try {
outputStream = new FileOutputStream(localFilePath);
byte[] buffer = new byte[1024];
int bytesRead = -1;
while ((bytesRead = inputStream.read(buffer)) != -1) {
outputStream.write(buffer, 0, bytesRead);
}
System.out.println("File downloaded: " + remoteFilePath);
} catch (IOException e) {
throw new RuntimeException("Failed to write to local file: " + localFilePath, e);
} finally {
if (outputStream != null) {
try {
outputStream.close();
} catch (IOException e) {
// ignore
}
}
if (inputStream != null) {
try {
inputStream.close();
} catch (IOException e) {
// ignore
}
}
}
sftpChannel.disconnect();
session.disconnect();
}
}
```
其中,`host` 为 SFTP 服务器地址,`port` 为 SFTP 服务器端口号,`username` 为登录用户名,`privateKeyPath` 为私钥文件的路径,`remoteFilePath` 为远程文件路径,`localFilePath` 为本地文件路径。
使用示例:
```java
public static void main(String[] args) throws Exception {
String host = "sftp.example.com";
int port = 22;
String username = "user";
String privateKeyPath = "/path/to/private/key";
String remoteFilePath = "/remote/file/path.txt";
String localFilePath = "/local/file/path.txt";
SFTPDownloader.downloadFile(host, port, username, privateKeyPath, remoteFilePath, localFilePath);
}
```
在实际使用时,需要将上述代码中的异常处理逻辑进行修改,以符合实际需求。
相关推荐
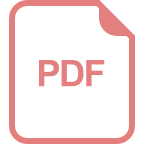
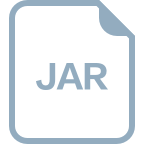














