在PHP页面生成一个2015-2030年的日历,要求突出当前日期,并可以通过点击←→按钮更改月份。并通过连接数据库,把数据库中日程安排内容输出到对应日期下。请生成完整代码
时间: 2023-12-28 09:04:54 浏览: 32
好的,以下是PHP页面生成日历并连接数据库的完整代码。请注意,这个代码只是一个示例,实际使用时需要根据具体情况进行修改和优化。
index.php:
```php
<!DOCTYPE html>
<html>
<head>
<title>Calendar</title>
<style>
table {
border-collapse: collapse;
margin: auto;
}
td {
border: 1px solid black;
padding: 10px;
text-align: center;
}
th {
padding: 10px;
text-align: center;
background-color: lightgray;
}
.current-day {
background-color: yellow;
}
</style>
</head>
<body>
<?php
// 获取当前月份和年份
if (isset($_GET['month']) && isset($_GET['year'])) {
$month = $_GET['month'];
$year = $_GET['year'];
} else {
$month = date('n');
$year = date('Y');
}
// 获取当前日期
$current_day = date('j');
// 计算当前月份的第一天和最后一天
$first_day = mktime(0, 0, 0, $month, 1, $year);
$last_day = mktime(0, 0, 0, $month + 1, 0, $year);
// 获取当前月份的天数和第一天是星期几
$num_days = date('j', $last_day);
$first_day_of_week = date('N', $first_day);
// 输出月份和年份
echo '<h2>' . date('F Y', $first_day) . '</h2>';
// 输出左箭头按钮,点击后返回上一个月份
echo '<a href="?month=' . ($month - 1) . '&year=' . $year . '"><</a>';
// 输出右箭头按钮,点击后进入下一个月份
echo '<a href="?month=' . ($month + 1) . '&year=' . $year . '">></a>';
// 输出日历表格
echo '<table>';
echo '<tr>';
echo '<th>Mon</th>';
echo '<th>Tue</th>';
echo '<th>Wed</th>';
echo '<th>Thu</th>';
echo '<th>Fri</th>';
echo '<th>Sat</th>';
echo '<th>Sun</th>';
echo '</tr>';
// 输出日历中的日期
$current_date = 1;
$blank = $first_day_of_week - 1;
$num_cells = ceil(($num_days + $blank) / 7) * 7;
for ($i = 0; $i < $num_cells; $i++) {
if ($i % 7 == 0) {
echo '<tr>';
}
if ($i < $blank || $i >= $num_days + $blank) {
echo '<td></td>';
} else {
if ($current_date == $current_day) {
echo '<td class="current-day">' . $current_date . '</td>';
} else {
echo '<td>' . $current_date . '</td>';
}
$current_date++;
}
if ($i % 7 == 6) {
echo '</tr>';
}
}
echo '</table>';
// 连接数据库,获取日程安排
$servername = "localhost";
$username = "username";
$password = "password";
$dbname = "myDB";
$conn = mysqli_connect($servername, $username, $password, $dbname);
if (!$conn) {
die("Connection failed: " . mysqli_connect_error());
}
$sql = "SELECT * FROM schedule WHERE month = $month AND year = $year";
$result = mysqli_query($conn, $sql);
if (mysqli_num_rows($result) > 0) {
while ($row = mysqli_fetch_assoc($result)) {
// 输出日程安排
echo '<p>' . $row['event'] . '</p>';
}
} else {
echo '<p>No schedule for this month.</p>';
}
mysqli_close($conn);
?>
</body>
</html>
```
在上面的代码中,我们首先获取当前月份和年份。如果用户通过左右箭头按钮切换了月份,则从URL参数中获取月份和年份。否则,使用PHP内置函数date()获取当前月份和年份。然后,我们计算当前月份的第一天和最后一天,以及当前月份的天数和第一天是星期几。接下来,我们使用一个for循环输出日历中的日期。如果日期是当前日期,则使用CSS样式“current-day”突出显示。然后,我们连接数据库,获取对应月份的日程安排,并将其输出到页面上。
请注意,这个代码中使用了MySQL数据库,你需要将$servername、$username、$password和$dbname替换为你自己的数据库信息。另外,这个代码只是示例代码,实际使用时需要对安全性和性能进行优化。
相关推荐
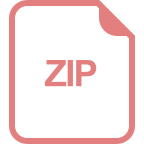
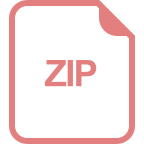














