1. 编写生成器函数,接收一个字符串,分别统计大写字母、小写字母、数字、其他字符的个数,并以元组的形式返回结果
时间: 2023-12-12 09:57:39 浏览: 84
好的,我可以帮您编写这个生成器函数。以下是代码实现:
```python
def count_chars(string):
upper_count = 0
lower_count = 0
digit_count = 0
other_count = 0
for char in string:
if char.isupper():
upper_count += 1
elif char.islower():
lower_count += 1
elif char.isdigit():
digit_count += 1
else:
other_count += 1
return (upper_count, lower_count, digit_count, other_count)
```
这个函数接收一个字符串作为参数,然后遍历字符串中的每个字符,判断它是大写字母、小写字母、数字还是其他字符,并分别记录它们的数量。最后,将这些数量打包成元组并返回。
您可以在代码中调用这个函数,例如:
```python
result = count_chars("Hello, World! 123")
print(result)
```
输出结果为:
```
(2, 8, 3, 3)
```
其中,元组的四个元素分别代表大写字母数量、小写字母数量、数字数量和其他字符数量。
相关问题
编写生成器函数,接收一个字符串,分别统计大写字母、小写字母、数字、其他字符的个数,并以元组的形式返回结果
好的,下面是生成器函数的代码实现:
```python
def count_chars(s):
upper_count = 0
lower_count = 0
digit_count = 0
other_count = 0
for c in s:
if c.isupper():
upper_count += 1
elif c.islower():
lower_count += 1
elif c.isdigit():
digit_count += 1
else:
other_count += 1
yield (upper_count, lower_count, digit_count, other_count)
```
这个函数接收一个字符串 `s`,然后用一个循环遍历字符串中的每个字符。根据字符的类型分别累加大写字母、小写字母、数字和其他字符的个数。最后用 `yield` 语句返回一个元组,包含各种字符的个数。注意这里使用了生成器函数,所以当你调用这个函数时,实际上只是得到一个生成器对象,需要使用 `next()` 函数来获取生成器的下一个值,或者使用 `for` 循环来迭代生成器对象。
编写生成器函数,接收一个字符串,分别统计大写字母、小写字母、数字、其他字符的个数,以元组的形式返回结果,返回的元组的第一关元素依次是字符串“大写字母”“小写字母”“数字”和“其他字符”,第二个元素是相应的字符个数。
好的,以下是实现该生成器函数的代码:
```python
def count_chars(s):
upper_count = 0
lower_count = 0
digit_count = 0
other_count = 0
for c in s:
if c.isupper():
upper_count += 1
elif c.islower():
lower_count += 1
elif c.isdigit():
digit_count += 1
else:
other_count += 1
return ("大写字母", upper_count), ("小写字母", lower_count), ("数字", digit_count), ("其他字符", other_count)
```
该函数接收一个字符串 `s`,然后遍历字符串中的每个字符,通过 `isupper()`、`islower()` 和 `isdigit()` 方法判断该字符是否为大写字母、小写字母和数字,如果都不是,则计入其他字符。最后将统计结果以元组的形式返回。
阅读全文
相关推荐
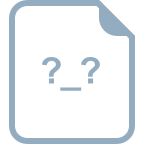
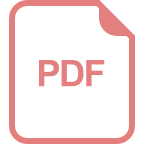
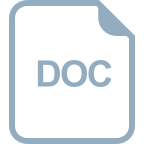


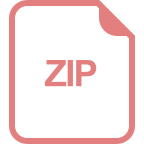
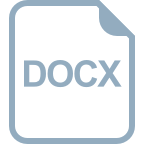
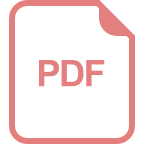
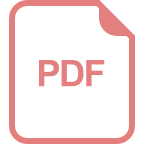
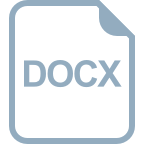
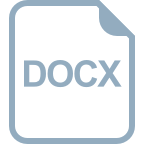
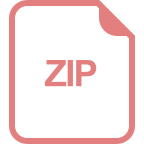