python写个数独破解器,代码实例
时间: 2023-06-06 22:04:20 浏览: 98
好的,以下是一个简单的数独破解器的Python代码实例:
```python
def solve_sudoku(board):
"""
Solve a Sudoku board using a backtracking algorithm.
:param board: 9x9 2D list of integers representing the Sudoku board
:return: True if the board is solvable, False otherwise
"""
# Find the next empty cell
row, col = find_empty_cell(board)
# Base case: if there are no empty cells, the board is solved
if row is None:
return True
# Try each number from 1 to 9 in the empty cell
for num in range(1, 10):
# Check if the number is valid in the given row, column, and box
if is_valid(board, row, col, num):
# If the number is valid, set it on the board
board[row][col] = num
# Recursively try to solve the board with the modified cell
if solve_sudoku(board):
return True
# If the modified cell leads to an unsolvable board, backtrack
board[row][col] = 0
# If none of the numbers from 1 to 9 are valid for the empty cell, backtrack
return False
def find_empty_cell(board):
"""
Find the next empty cell on the board.
:param board: 9x9 2D list of integers representing the Sudoku board
:return: (row, col) tuple of the next empty cell, or (None, None) if the board is full
"""
for row in range(9):
for col in range(9):
if board[row][col] == 0:
return (row, col)
return (None, None)
def is_valid(board, row, col, num):
"""
Check whether the given number is valid in the given row, column, and box.
:param board: 9x9 2D list of integers representing the Sudoku board
:param row: row index of the cell being checked
:param col: column index of the cell being checked
:param num: the number being checked for validity
:return: True if the number is valid, False otherwise
"""
# Check row
if num in board[row]:
return False
# Check column
if num in [board[i][col] for i in range(9)]:
return False
# Check box
box_row, box_col = 3 * (row // 3), 3 * (col // 3)
if num in [board[r][c] for r in range(box_row, box_row + 3) for c in range(box_col, box_col + 3)]:
return False
return True
```
你可以调用该函数并传入一个9x9的矩阵表示数独问题。该函数将返回True如果数独问题有解,否则返回False。如果问题有解,解将被直接修改在传入的矩阵中。
阅读全文
相关推荐
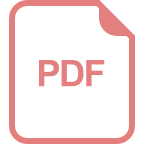
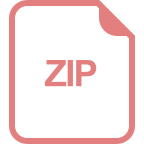
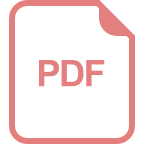
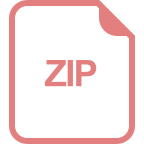
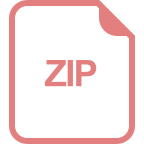
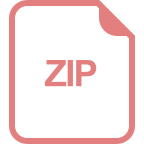
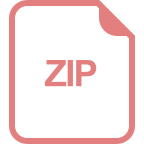
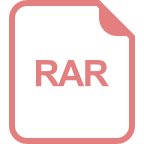
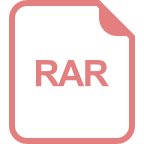
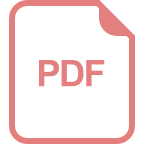