encoding = chardet.detect(html_table)['encoding'] soup = BeautifulSoup(html_table, 'html.parser', from_encoding=encoding)用這樣的方式改變編碼格式時報這樣的錯是什麽原因呢,該怎麽解決TypeError: Expected object of type bytes or bytearray, got: <class 'str'>
时间: 2023-12-17 12:04:45 浏览: 45
这个错误的原因是`chardet.detect()`函数返回的是一个字典,其中包含了网页编码的信息,而不是一个字符串。因此,你需要对`html_table`进行编码,然后再将其传递给BeautifulSoup。
你可以使用如下代码进行修改:
```python
import chardet
# 检测编码并编码字符串
encoding = chardet.detect(html_table.encode())['encoding']
html_table_encoded = html_table.encode(encoding)
# 将编码过的字符串传递给BeautifulSoup
soup = BeautifulSoup(html_table_encoded, 'html.parser', from_encoding=encoding)
```
在这个代码中,我们首先使用`encode()`方法将`html_table`字符串编码为字节串。然后,使用`chardet.detect()`函数检测字节串的编码格式,并返回一个字典。最后,我们将编码过的字节串和编码信息传递给BeautifulSoup,以正确地解析网页内容。
相关问题
encoding = chardet.detect(fileinfo.filename)[encoding],报错Expected object of type bytes or bytearray, got: <class 'str'>
这个错误提示告诉我们,`fileinfo.filename` 应该是一个字节序列(bytes 或 bytearray),但它实际上是字符串(str)。因此,需要将字符串转换为字节序列,可以使用字符串的 `encode` 方法来实现。例如,将字符串转换为 UTF-8 编码的字节序列可以这样写:`fileinfo.filename.encode('utf-8')`。修改代码如下:
```python
encoding = chardet.detect(fileinfo.filename.encode('utf-8'))['encoding']
```
这样就可以避免这个错误了。
import chardet s = b'\xc4\xe3\xba\xc3' encoding = chardet.detect(s)['encoding'] s = s.decode(encoding)这串代码是什么意思帮我打一下注释
```
# 导入 chardet 库
import chardet
# 定义一个二进制字节串
s = b'\xc4\xe3\xba\xc3'
# 使用 chardet.detect() 探测 s 的编码方式,并将编码方式赋值给 encoding 变量
encoding = chardet.detect(s)['encoding']
# 将 s 解码为字符串,解码方式为 encoding 变量的值
s = s.decode(encoding)
```
这段代码的功能是:探测二进制字节串 s 的编码方式,并将其解码为字符串。其中,chardet.detect() 方法可以自动探测字节串的编码方式,返回一个字典,其中包含了编码方式等信息。最终,通过 decode() 方法将字节串解码为字符串。
相关推荐
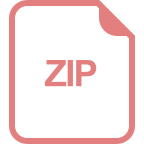
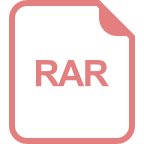
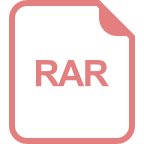













