ffmepg rtsp取流C编程
时间: 2023-11-02 13:06:42 浏览: 38
ffmpeg是一个非常强大的开源软件库,可以用来处理视频和音频,同时也提供了实时流处理的功能。下面是一些基本的C代码,可以使用ffmpeg来从RTSP流中获取视频帧。
1. 初始化ffmpeg
在使用ffmpeg之前,需要先初始化ffmpeg的环境。
```c
av_register_all(); // 注册所有的AVCodec和AVFormat
avformat_network_init(); //初始化网络流处理
```
2. 打开RTSP流
使用avformat_open_input函数打开RTSP流。
```c
AVFormatContext *pFormatCtx = avformat_alloc_context();
if (avformat_open_input(&pFormatCtx, rtspUrl, NULL, NULL) != 0) {
printf("Couldn't open input stream.\n");
return -1;
}
```
3. 查找流信息
使用avformat_find_stream_info函数查找流信息。
```c
if (avformat_find_stream_info(pFormatCtx, NULL) < 0) {
printf("Couldn't find stream information.\n");
return -1;
}
```
4. 查找视频流
使用av_find_best_stream函数查找视频流。
```c
int video_stream_index = av_find_best_stream(pFormatCtx, AVMEDIA_TYPE_VIDEO, -1, -1, NULL, 0);
if (video_stream_index < 0) {
printf("Couldn't find video stream.\n");
return -1;
}
```
5. 获取视频解码器
使用avcodec_find_decoder函数查找视频解码器。
```c
AVCodecContext *pCodecCtx = pFormatCtx->streams[video_stream_index]->codec;
AVCodec *pCodec = avcodec_find_decoder(pCodecCtx->codec_id);
if (pCodec == NULL) {
printf("Couldn't find video decoder.\n");
return -1;
}
if (avcodec_open2(pCodecCtx, pCodec, NULL) < 0) {
printf("Couldn't open video decoder.\n");
return -1;
}
```
6. 读取视频帧
使用av_read_frame函数读取视频帧。
```c
AVPacket packet;
while (av_read_frame(pFormatCtx, &packet) >= 0) {
if (packet.stream_index == video_stream_index) {
AVFrame *pFrame = av_frame_alloc();
int got_picture = 0;
if (avcodec_decode_video2(pCodecCtx, pFrame, &got_picture, &packet) < 0) {
printf("Failed to decode video frame.\n");
return -1;
}
if (got_picture) {
//处理视频帧
}
av_frame_free(&pFrame);
}
av_packet_unref(&packet);
}
```
7. 释放资源
使用avcodec_close和avformat_close_input函数释放资源。
```c
avcodec_close(pCodecCtx);
avformat_close_input(&pFormatCtx);
```
以上就是使用ffmpeg从RTSP流中获取视频帧的基本流程。需要注意的是,如果要实现实时的视频流处理,需要在循环中添加一些额外的逻辑来控制帧率和处理速度,以避免过多的延迟和缓存。
相关推荐
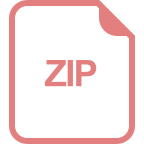
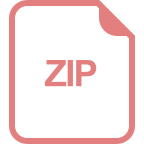
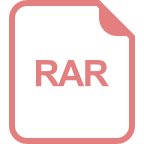














