怎么创建 AttributeSet
时间: 2023-09-08 16:05:29 浏览: 58
### 回答1:
在Android中,可以通过以下代码来创建 AttributeSet:
```
XmlPullParser parser = context.getResources().getXml(R.xml.my_attributes);
AttributeSet attributes = Xml.asAttributeSet(parser);
```
其中,R.xml.my_attributes是你定义的XML文件的资源ID,可以在XML文件中定义一组自定义属性,例如:
```
<?xml version="1.0" encoding="utf-8"?>
<resources>
<declare-styleable name="MyView">
<attr name="my_custom_attribute" format="string" />
</declare-styleable>
</resources>
```
这个XML文件定义了一个名为MyView的自定义View,在该View中可以使用一个名为my_custom_attribute的自定义属性。在创建 AttributeSet 的时候,可以将该XML文件作为参数传入,这样就可以创建一个包含自定义属性的 AttributeSet 对象了。
### 回答2:
创建 AttributeSet 可以通过以下步骤进行。
首先,我们需要在代码中创建一个新的 AttributeSet 对象。可以通过使用 Context 的 obtainStyledAttributes() 方法来实现。此方法返回一个 TypedArray 对象,其中包含了 AttributeSet 的值。
接下来,我们需要从我们已经创建的 AttributeSet 对象中获取我们想要的属性值。可以使用 TypedArray 的各种方法来获取属性值,比如 getBoolean()、getDimension()、getString() 等。我们需要根据我们需要的属性类型来选择正确的方法。
在获取完所有属性值后,我们可以使用 TypedArray 的 recycle() 方法来释放已经不再需要的资源。这个方法非常重要,因为它可以帮助我们防止内存泄漏。
最后,我们可以根据获取到的属性值调整我们的视图或其他逻辑。比如,如果我们获取到一个布尔值属性,我们可以根据其值来显示或隐藏某个控件;如果我们获取到一个字符串属性,我们可以将其设置为 TextView 的文本内容。
总的来说,创建 AttributeSet 的过程需要使用 Context 的 obtainStyledAttributes() 方法来获取 TypedArray 对象,然后使用 TypedArray 的各种方法来获取属性值,最后使用 recycle() 方法来释放资源。这样我们就能够得到一个有效的 AttributeSet 对象,并根据其属性值来做进一步的处理。
### 回答3:
在Android开发中,我们可以使用 AttributeSet 来创建自定义 View 的属性集合。下面我将简要说明如何创建 AttributeSet。
1. 创建 attrs.xml 文件:首先,在 res/values 文件夹下创建一个新的 xml 文件,命名为 attrs.xml。这个文件将用于定义自定义属性的名称和类型。
2. 定义自定义属性:在 attrs.xml 文件中,我们可以使用 <declare-styleable> 标签来定义一个新的属性集合。在该标签内,可以使用 <attr> 标签来定义具体的属性。例如:
```
<resources>
<declare-styleable name="CustomView">
<attr name="customAttribute1" format="string" />
<attr name="customAttribute2" format="integer" />
</declare-styleable>
</resources>
```
在上面的代码中,我们定义了一个名为 CustomView 的属性集合,并在该属性集合中添加了两个自定义属性,分别是 customAttribute1 和 customAttribute2,其类型分别为字符串和整数。
3. 使用 AttributeSet:在自定义 View 的构造方法中,我们可以使用 AttributeSet 参数来获取传入 View 的属性集合。例如:
```
public CustomView(Context context, AttributeSet attrs) {
super(context, attrs);
TypedArray typedArray = context.obtainStyledAttributes(attrs, R.styleable.CustomView);
String customAttribute1 = typedArray.getString(R.styleable.CustomView_customAttribute1);
int customAttribute2 = typedArray.getInt(R.styleable.CustomView_customAttribute2, defaultValue);
// 使用获取到的属性值进行相关操作
typedArray.recycle();
}
```
在上面的代码中,我们通过 context.obtainStyledAttributes 方法获取了属性集合的 TypedArray 对象。然后,通过指定自定义属性的名称来获取对应的属性值。最后,记得使用 typedArray.recycle() 方法来回收 TypedArray 对象。
总结:通过创建 attrs.xml 文件并定义自定义属性,然后在自定义 View 的构造方法中使用 AttributeSet 参数来获取属性集合的 TypedArray 对象,我们就可以轻松地创建和使用 AttributeSet。
相关推荐
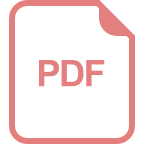
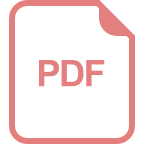
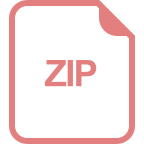














