用java语言,访问SQLServer或者MySQL数据库,使用 JDBC API构建应用程序,对学生信息表(student)执行以下各种数据库操作:查询表、在表中插入行、在表中更新行、从表中删除行。要求使用PreparedStatement对象查询并修改数据。
时间: 2024-03-24 19:39:37 浏览: 35
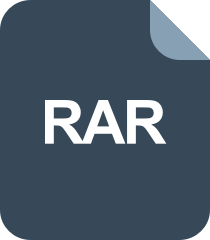
基于JAVA超市综合管理信息系统的毕业设计,数据使用JDBC技术实现,与MySQL数据库的连接和数据的查询、插入、更新、删除操作
好的,这是一个关于Java语言连接SQLServer或MySQL数据库的问题,并对学生信息表执行一些基本的CRUD操作。下面是一个简单的示例代码,演示了如何使用JDBC API连接数据库和执行操作:
```java
import java.sql.*;
public class StudentDatabase {
// JDBC driver name and database URL
static final String JDBC_DRIVER = "com.mysql.jdbc.Driver";
static final String DB_URL = "jdbc:mysql://localhost/STUDENT";
// Database credentials
static final String USER = "username";
static final String PASS = "password";
public static void main(String[] args) {
Connection conn = null;
PreparedStatement stmt = null;
try {
// Register JDBC driver
Class.forName(JDBC_DRIVER);
// Open a connection
System.out.println("Connecting to database...");
conn = DriverManager.getConnection(DB_URL, USER, PASS);
// Query the student table
System.out.println("Querying student table...");
stmt = conn.prepareStatement("SELECT id, name, age FROM student");
ResultSet rs = stmt.executeQuery();
// Process the result set
while (rs.next()) {
int id = rs.getInt("id");
String name = rs.getString("name");
int age = rs.getInt("age");
System.out.println("ID: " + id + ", Name: " + name + ", Age: " + age);
}
// Insert a new student
System.out.println("Inserting a new student...");
stmt = conn.prepareStatement("INSERT INTO student (name, age) VALUES (?, ?)");
stmt.setString(1, "John");
stmt.setInt(2, 20);
int rowsInserted = stmt.executeUpdate();
System.out.println(rowsInserted + " row(s) inserted.");
// Update a student's age
System.out.println("Updating a student's age...");
stmt = conn.prepareStatement("UPDATE student SET age = ? WHERE name = ?");
stmt.setInt(1, 21);
stmt.setString(2, "John");
int rowsUpdated = stmt.executeUpdate();
System.out.println(rowsUpdated + " row(s) updated.");
// Delete a student
System.out.println("Deleting a student...");
stmt = conn.prepareStatement("DELETE FROM student WHERE name = ?");
stmt.setString(1, "John");
int rowsDeleted = stmt.executeUpdate();
System.out.println(rowsDeleted + " row(s) deleted.");
} catch (SQLException se) {
// Handle errors for JDBC
se.printStackTrace();
} catch (Exception e) {
// Handle errors for Class.forName
e.printStackTrace();
} finally {
// Close resources
try {
if (stmt != null) stmt.close();
} catch (SQLException se2) {
} // nothing we can do
try {
if (conn != null) conn.close();
} catch (SQLException se) {
se.printStackTrace();
}
System.out.println("Goodbye!");
}
}
}
```
这个示例代码使用了MySQL数据库,你需要将代码中的URL、用户名和密码替换成你自己的。另外,你需要将代码中的表名、列名和数据类型替换成你自己的学生信息表的结构。
这个示例代码包含了查询表、插入行、更新行和删除行四种操作,都使用了PreparedStatement对象,可以有效地防止SQL注入攻击。你可以根据自己的需要添加更多的操作。
阅读全文
相关推荐
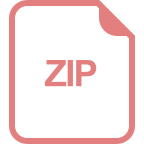

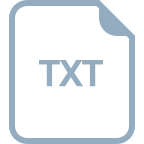
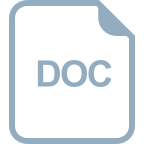

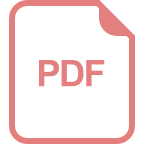
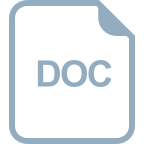
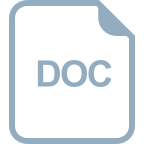
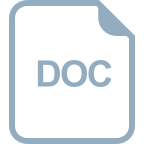
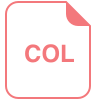







