base64转blob vue
时间: 2023-07-29 13:07:05 浏览: 47
可以使用以下代码将 base64 字符串转换为 Blob 对象:
```javascript
// 将 base64 字符串转换为 Blob 对象
function base64ToBlob(base64Str) {
const parts = base64Str.split(';base64,');
const contentType = parts[0].split(':')[1];
const raw = window.atob(parts[1]);
const rawLength = raw.length;
const uInt8Array = new Uint8Array(rawLength);
for (let i = 0; i < rawLength; ++i) {
uInt8Array[i] = raw.charCodeAt(i);
}
return new Blob([uInt8Array], { type: contentType });
}
```
在 Vue 中,可以在组件的方法中调用该函数,例如:
```vue
<template>
<div>
<input type="file" ref="fileInput" @change="handleFileChange">
</div>
</template>
<script>
export default {
methods: {
handleFileChange() {
const file = this.$refs.fileInput.files[0];
const reader = new FileReader();
reader.onload = () => {
const base64Str = reader.result;
const blob = this.base64ToBlob(base64Str);
console.log(blob);
// 在这里可以将 blob 对象上传到服务器或者进行其他操作
};
reader.readAsDataURL(file);
},
// 将 base64 字符串转换为 Blob 对象
base64ToBlob(base64Str) {
const parts = base64Str.split(';base64,');
const contentType = parts[0].split(':')[1];
const raw = window.atob(parts[1]);
const rawLength = raw.length;
const uInt8Array = new Uint8Array(rawLength);
for (let i = 0; i < rawLength; ++i) {
uInt8Array[i] = raw.charCodeAt(i);
}
return new Blob([uInt8Array], { type: contentType });
},
},
};
</script>
```
在上述代码中,我们首先使用 `<input type="file">` 元素让用户选择文件,并在 `handleFileChange` 方法中读取文件内容并将其转换为 base64 字符串。然后,我们调用 `base64ToBlob` 方法将 base64 字符串转换为 Blob 对象,并可以将其上传到服务器或者进行其他操作。
相关推荐
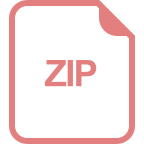
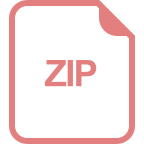














