#include <stdio.h> #include <____1_____> int main() { char s[81]; int i; _____2_____; for(i=0;____3_____;i++) { if(isupper(s[i])) s[i]=s[i]+32; else if(islower(s[i])) s[i]=s[i]-32; if(____4____) s[i]='_'; } puts(s); }
时间: 2024-03-02 12:54:36 浏览: 73
这段代码中有 4 处需要填空:
```c
#include <stdio.h>
#include <ctype.h>
int main() {
char s[81];
int i;
gets(s);
for(i=0;s[i]!='\0';i++) {
if(isupper(s[i]))
s[i]=s[i]+32;
else if(islower(s[i]))
s[i]=s[i]-32;
if(s[i]==' ')
s[i]='_';
}
puts(s);
return 0;
}
```
第一处需要填空的是头文件 `ctype.h`,包含了一些字符分类函数,例如 `isupper` 和 `islower`。
第二处需要填空的是输入字符串 `s`,应该使用 `gets` 函数,这样可以读入包含空格的字符串。
第三处需要填空的是 `for` 循环的终止条件,应该循环直到遇到字符串结束符 `\0`,即 `s[i]!='\0'`。
第四处需要填空的是空格字符 `' '` 的判断,应该使用字符字面量 `' '`,即 `s[i]==' '`。
运行程序后,程序将会提示输入一个字符串,然后将其中的大小写字母互换并将空格替换为下划线,并输出转换后的字符串。
相关问题
#include <stdio.h> #include <____1____> int main() { char s[81];int i; gets(s); for(___2___;i<strlen(s);) if(s[i]=='c') strcpy(____3____); ___4___ i++; puts(s); }填空
好的,下面是代码填空的答案:
```c
#include <stdio.h>
#include <string.h> //填空1
int main() {
char s[81];
int i = 0; //填空2
gets(s);
for(;i<strlen(s);)
if(s[i]=='c')
strcpy(s+i,"cccc"); //填空3
else
i++; //填空4
puts(s);
return 0;
}
```
其中,第一个空需要包含字符串操作函数,此处是 `string.h`。第二个空需要将计数器 i 的初始值赋为 0,此处是 `int i = 0;`。第三个空需要在字符串 s 中从 i 开始插入 "cccc",此处是 `strcpy(s+i,"cccc");`。第四个空需要将计数器 i 加 1,即跳过已经替换过的字符,此处是 `i++;`。
完善以下程序#include <stdio.h> #include <string.h> #include <___1___> void f(char *s) { ___ 2___; while(s[i]!='\0') if(isdigit(s[i])) ____3____(s+i,s+i+1); ___4___ i++; } int main() { char str[80]; gets(str); f(str); puts(str); }
完善以下程序:
```c
#include <stdio.h>
#include <string.h>
#include <ctype.h>
void f(char *s) {
int i = 0;
while(s[i] != '\0') {
if(isdigit(s[i])) {
memmove(s+i, s+i+1, strlen(s+i+1)+1);
}
else {
i++;
}
}
}
int main() {
char str[80];
gets(str);
f(str);
puts(str);
return 0;
}
```
在这个程序中,函数 `f` 的作用是删除字符串中的所有数字字符。具体地,它遍历字符串中的每一个字符,如果它是数字字符,则将其从字符串中移除。
在实现中,我们使用了 `isdigit` 函数来判断一个字符是否是数字字符,使用了 `memmove` 函数来移动字符串中的字符。在移动字符时,我们使用了 `strlen` 函数来计算需要移动的字符数,并在最后添加一个空字符,以确保字符串的结尾正确。
阅读全文
相关推荐
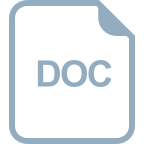
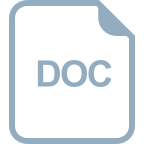
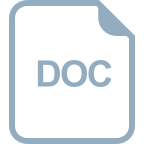














