可以向我展示一段包含unix 高级编程知识点的代码,要是使用open()、close()、read()、write()、lseek()这些接口,并依次展示接口的所有用法
时间: 2023-03-01 19:29:28 浏览: 99
以下是一段使用Unix的高级编程知识点的代码:#include <stdio.h>
#include <unistd.h>
#include <sys/types.h>int main()
{
int fd;
char buf[50]; // 使用open()函数打开文件
fd = open("test.txt", O_RDWR);
if (fd == -1) {
perror("open error");
return -1;
} // 使用read()函数读取文件
read(fd, buf, 50);
printf("buf: %s\n", buf); // 使用lseek()函数定位文件指针
lseek(fd, 5, SEEK_SET); // 使用write()函数写入文件
write(fd, "abcde", 5); // 使用close()函数关闭文件
close(fd); return 0;
}
相关问题
可以向我展示一段包含unix 高级编程知识点的代码,要求使用open()、close()、read()、write()、lseek(),并展示并解释这些函数的所有知识点
好的,以下是一个简单的代码示例,它使用了 unix 高级编程中的 open()、close()、read()、write()、lseek() 函数:
```
#include <fcntl.h>
#include <unistd.h>
#include <stdio.h>
int main()
{
// 使用 open() 函数打开文件
int fd = open("file.txt", O_RDWR | O_CREAT, 0644);
if (fd == -1) {
perror("open");
return 1;
}
// 使用 write() 函数写入内容到文件
const char *content = "Hello, Unix Advanced Programming!";
if (write(fd, content, strlen(content)) == -1) {
perror("write");
close(fd);
return 1;
}
// 使用 lseek() 函数调整读写指针的位置
if (lseek(fd, 0, SEEK_SET) == -1) {
perror("lseek");
close(fd);
return 1;
}
// 使用 read() 函数读取文件内容
char buffer[1024];
int n = read(fd, buffer, sizeof buffer);
if (n == -1) {
perror("read");
close(fd);
return 1;
}
// 打印读取的内容
buffer[n] = '\0';
printf("Read from file: %s\n", buffer);
// 使用 close() 函数关闭文件
if (close(fd) == -1) {
perror("close");
return 1;
}
return 0;
}
```
关于这些函数的详细知识点:
- open():此函数用于打开文件,它需要指定文件名和打开模式,返回一个整数文件描述符(file descriptor),用于之后的读写操作。
- close():此函数用于关闭文件,它需要指定文件描述符,在完成所有读写操作后应该关闭文件以
可以向我展示一段包含unix 高级编程知识点的代码,要求使用open()、close()、read()、write()、lseek()这些函数的各种类型参数,并付上知识点详细解释
好的,以下是一段包含unix高级编程知识点的代码:
```
#include <fcntl.h>
#include <unistd.h>
#include <stdio.h>
#include <string.h>
#include <errno.h>
#define BUFSIZE 512
int main(int argc, char *argv[]) {
int fd;
ssize_t nread;
char buf[BUFSIZE];
off_t curr_pos;
// 使用O_RDONLY只读方式打开文件
if ((fd = open(argv[1], O_RDONLY)) == -1) {
perror("open");
return 1;
}
// 读取文件内容并输出
while ((nread = read(fd, buf, BUFSIZE)) > 0) {
if (write(1, buf, nread) == -1) {
perror("write");
return 1;
}
}
if (nread == -1) {
perror("read");
return 1;
}
// 获取当前文件读写位置
if ((curr_pos = lseek(fd, 0, SEEK_CUR)) == -1) {
perror("lseek");
return 1;
}
printf("Current position: %lld\n", curr_pos);
// 关闭文件
if (close(fd) == -1) {
perror("close");
return 1;
}
return 0;
}
```
该代码演示了unix中一些常用的高级编程函数的用法:
- open():该函数用于打开一个文件,它接受两个参数:文件路径和打开方式。这里使用了O_RDONLY只读方式打开文件。
- read():该函数用于从已打开的文件中读取数据,它接受三个参数:文件描述符、缓存区和要读取的字节数。该函数每次读取一定量的数据,并将读到的数据放入缓存区
阅读全文
相关推荐


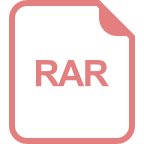
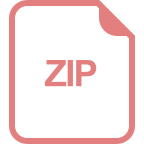
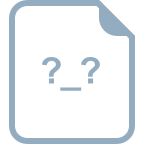