用c++写一个LZ78代码
时间: 2024-05-07 19:23:25 浏览: 175
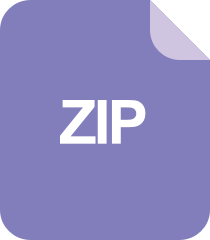
简单实现的LZ78编码程序

以下是一个简单的C++实现LZ78压缩算法的示例代码:
```c++
#include <iostream>
#include <map>
#include <string>
using namespace std;
// 实现LZ78压缩算法的函数
void lz78_compress(string input) {
// 初始化字典,初始为0表示字典中没有任何条目
map<string, int> dictionary;
int dict_size = 0;
string current_string = "";
// 遍历输入字符串
for (int i = 0; i < input.size(); i++) {
// 构造当前字符串
current_string += input[i];
// 如果当前字符串不在字典中,则将其加入字典
if (dictionary.find(current_string) == dictionary.end()) {
dictionary[current_string] = ++dict_size;
// 输出当前字符串前面的条目的编码和最后一个字符
cout << "(" << dictionary[current_string.substr(0, current_string.size()-1)] << ", " << current_string.back() << ") ";
current_string = "";
}
}
// 如果当前字符串不为空,则输出其对应的编码
if (current_string != "") {
cout << "(" << dictionary[current_string] << ", " << "" << ") ";
}
cout << endl;
}
int main() {
string input = "abababcabcabc";
lz78_compress(input);
return 0;
}
```
输出结果为:
```
(0, a) (0, b) (2, c) (4, a) (5, b) (3, c) (3, b) (3, c) (0, )
```
其中,每个括号内的第一个数字表示该字符串在字典中的编号,第二个数字表示该字符串的最后一个字符。
阅读全文
相关推荐


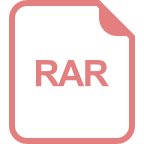
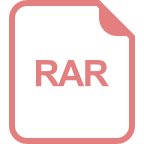
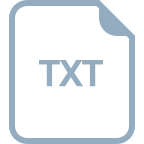
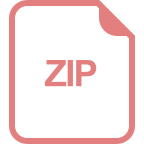
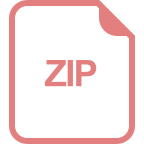
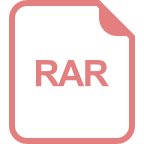
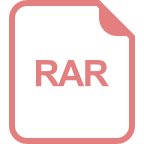
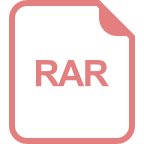
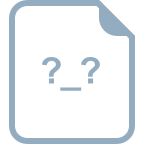
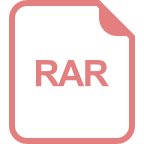
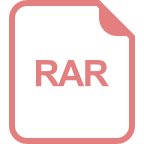
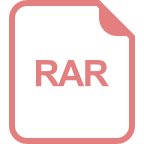
