vue3 服务端渲染页面title和meta标签,不引入外部第三方库,给出所有可能的方法
时间: 2024-03-09 16:46:15 浏览: 206
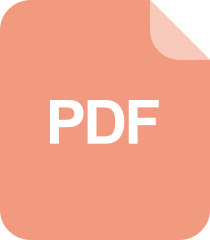
vue中动态设置meta标签和title标签的方法
在 Vue3 中,你可以通过以下方法在服务端渲染时设置页面的 title 和 meta 标签:
1. 使用 `renderToString` 方法的第二个参数 context 来设置 title 和 meta 标签。
```javascript
import { createApp } from 'vue';
import App from './App.vue';
const app = createApp(App);
app.mount('#app');
// 在服务端渲染时设置 title 和 meta 标签
const context = { title: 'My Page', meta: { description: 'This is my page', keywords: 'vue, ssr, meta' } };
const html = await renderToString(app, context);
```
在模板中使用以下代码来渲染 title 和 meta 标签:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>{{ context.title }}</title>
<meta name="description" :content="context.meta.description">
<meta name="keywords" :content="context.meta.keywords">
</head>
<body>
<div id="app">{{ html }}</div>
</body>
</html>
```
2. 在组件的 `setup` 方法中使用 `onMounted` 钩子来设置 title 和 meta 标签。
```html
<template>
<div>
<h1>Hello World</h1>
</div>
</template>
<script>
import { onMounted } from 'vue';
export default {
setup() {
onMounted(() => {
document.title = 'My Page';
const description = document.createElement('meta');
description.setAttribute('name', 'description');
description.setAttribute('content', 'This is my page');
document.head.appendChild(description);
const keywords = document.createElement('meta');
keywords.setAttribute('name', 'keywords');
keywords.setAttribute('content', 'vue, ssr, meta');
document.head.appendChild(keywords);
});
},
};
</script>
```
这种方法需要注意的是,服务端渲染时 `document` 对象是不存在的,因此需要判断是否在浏览器环境下运行。
3. 在使用 `createApp` 创建应用程序实例时,传入第二个参数 options 来设置 title 和 meta 标签。
```javascript
import { createApp } from 'vue';
import App from './App.vue';
const app = createApp(App, {
title: 'My Page',
meta: [
{ name: 'description', content: 'This is my page' },
{ name: 'keywords', content: 'vue, ssr, meta' },
],
});
app.mount('#app');
```
在模板中使用以下代码来渲染 title 和 meta 标签:
```html
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8">
<title>{{ options.title }}</title>
<meta name="description" :content="options.meta.find((meta) => meta.name === 'description').content">
<meta name="keywords" :content="options.meta.find((meta) => meta.name === 'keywords').content">
</head>
<body>
<div id="app">{{ html }}</div>
</body>
</html>
```
这种方法需要注意的是,在组件的 `setup` 方法中不能通过 `document.title` 等方式修改标题和 meta 标签,因为这些标签已经在应用程序实例创建时就被设置好了。
阅读全文
相关推荐
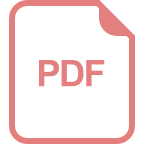
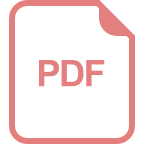















