so now, i want to implement a process to create a command named mytime using fork() to run another process, how can i do that
时间: 2024-04-30 16:21:04 浏览: 78
Sure, I can help you with that.
First, let me clarify that `fork()` is a system call that creates a new process by duplicating the calling process. So, in order to create a new process to run the `mytime` command, you need to do the following:
1. Use `fork()` to create a child process.
2. In the child process, use `execvp()` function to execute the `mytime` command.
3. In the parent process, wait for the child process to complete using `waitpid()` function.
Here's a code example:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <sys/wait.h>
int main() {
pid_t pid;
int status;
pid = fork();
if (pid == 0) {
// Child process
char *args[] = {"mytime", NULL};
execvp(args[0], args);
exit(EXIT_FAILURE); // execvp only returns if an error occurs
} else if (pid < 0) {
// Error occurred while forking
fprintf(stderr, "Failed to fork process\n");
exit(EXIT_FAILURE);
} else {
// Parent process
waitpid(pid, &status, 0);
printf("Child process exited with status: %d\n", status);
}
return 0;
}
```
In this example, the `fork()` function is used to create a child process. If `fork()` returns a value less than zero, an error occurred and the program exits. If `fork()` returns zero, then we are in the child process and we call `execvp()` to execute the `mytime` command. If `fork()` returns a positive value, then we are in the parent process and we wait for the child process to complete using `waitpid()` function.
I hope this helps! Let me know if you have any questions.
相关推荐
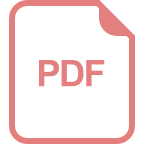
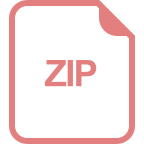
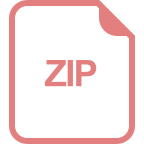
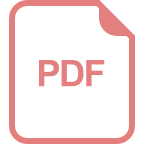
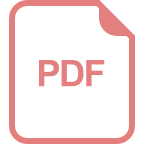
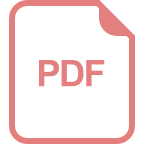
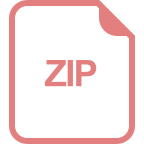
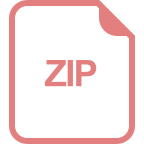
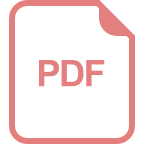
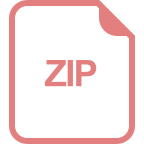
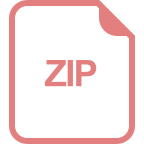
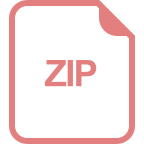
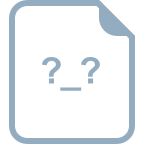
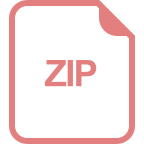
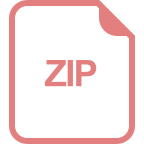
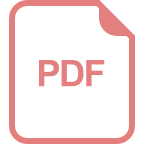