linux设计两个程序:有名管道的读程序和写程序,要求利用有名管道实现聊天程序,每次发言后自动在后面增加当前系统时间。增加结束字符,比如最后输入“886”后结束进程
时间: 2023-06-24 07:08:28 浏览: 140
以下是一个简单的基于有名管道的聊天程序的示例,包含读程序和写程序:
写程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <string.h>
#include <time.h>
#define PIPE_NAME "/tmp/chat_pipe"
int main() {
int fd;
char input[100];
time_t now;
struct tm *local_time;
char time_str[80];
// 打开有名管道
fd = open(PIPE_NAME, O_WRONLY);
if (fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
while (1) {
printf("请输入聊天内容:");
fgets(input, sizeof(input), stdin);
// 获取当前时间,格式化为字符串
now = time(NULL);
local_time = localtime(&now);
strftime(time_str, sizeof(time_str), "[%Y-%m-%d %H:%M:%S] ", local_time);
// 将聊天内容和时间字符串写入管道
write(fd, time_str, strlen(time_str));
write(fd, input, strlen(input));
// 如果输入了结束字符,则结束程序
if (strncmp(input, "886", 3) == 0) {
break;
}
}
// 关闭管道
close(fd);
return 0;
}
```
读程序:
```c
#include <stdio.h>
#include <stdlib.h>
#include <unistd.h>
#include <fcntl.h>
#include <string.h>
#define PIPE_NAME "/tmp/chat_pipe"
int main() {
int fd;
char buffer[1024];
int n;
// 创建有名管道
if (mkfifo(PIPE_NAME, 0666) == -1) {
perror("mkfifo");
exit(EXIT_FAILURE);
}
// 打开有名管道
fd = open(PIPE_NAME, O_RDONLY);
if (fd == -1) {
perror("open");
exit(EXIT_FAILURE);
}
while (1) {
// 从管道中读取数据
n = read(fd, buffer, sizeof(buffer));
if (n <= 0) {
break;
}
// 打印聊天内容
buffer[n] = '\0';
printf("%s", buffer);
// 如果读到了结束字符,则结束程序
if (strncmp(buffer + n - 4, "886\n", 4) == 0) {
break;
}
}
// 关闭管道
close(fd);
// 删除有名管道
unlink(PIPE_NAME);
return 0;
}
```
使用方法:
1. 在终端中先运行读程序,再运行写程序。
2. 写程序会提示输入聊天内容,输入后会自动在前面加上当前系统时间并写入管道。
3. 读程序会从管道中读取数据,并打印出来。如果读到了结束字符,则结束程序。
4. 注意,写程序和读程序需要在不同的终端中运行。
阅读全文
相关推荐
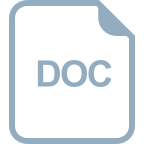

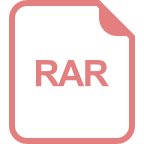
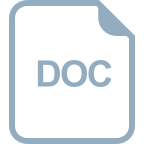
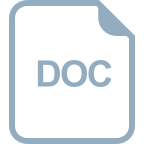
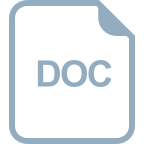
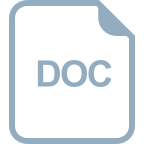
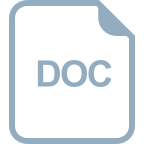
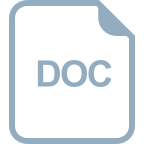
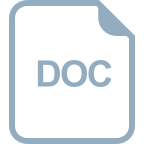
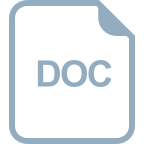
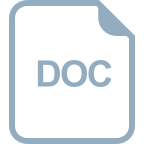
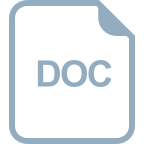
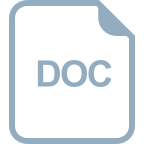
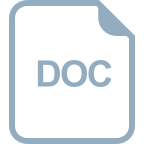
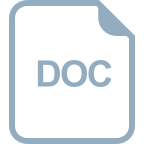
