医生讲座签到功能实现代码实现 基于springboot实现
时间: 2023-12-15 22:52:03 浏览: 133
以下是一个基于 Spring Boot 实现的医生讲座签到功能的代码实现:
首先,在 `pom.xml` 文件中添加以下依赖:
```xml
<dependencies>
<!-- Spring Boot Web Starter -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-web</artifactId>
</dependency>
<!-- Spring Boot Data JPA Starter -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<!-- MySQL Connector/J -->
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
<!-- Thymeleaf -->
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-thymeleaf</artifactId>
</dependency>
</dependencies>
```
然后,在 `application.properties` 文件中添加以下配置:
```
# 数据库配置
spring.datasource.url=jdbc:mysql://localhost:3306/sign-in?useSSL=false&serverTimezone=UTC
spring.datasource.username=root
spring.datasource.password=123456
spring.datasource.driver-class-name=com.mysql.jdbc.Driver
# JPA 配置
spring.jpa.show-sql=true
spring.jpa.hibernate.ddl-auto=update
spring.jpa.properties.hibernate.dialect=org.hibernate.dialect.MySQL5InnoDBDialect
spring.jpa.properties.hibernate.format_sql=true
```
接下来,创建一个 `Lecture` 实体类,表示医生讲座:
```java
@Entity
@Table(name = "lecture")
public class Lecture {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false)
private String title;
@Column(nullable = false)
private String speaker;
@Column(nullable = false)
private LocalDateTime startTime;
@Column(nullable = false)
private LocalDateTime endTime;
@OneToMany(mappedBy = "lecture", cascade = CascadeType.ALL)
private Set<Attendance> attendanceSet;
// 省略 getter/setter 方法
}
```
其中,`Attendance` 实体类表示签到记录:
```java
@Entity
@Table(name = "attendance")
public class Attendance {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@ManyToOne(fetch = FetchType.LAZY)
@JoinColumn(name = "lecture_id")
private Lecture lecture;
@Column(nullable = false)
private LocalDateTime signInTime;
// 省略 getter/setter 方法
}
```
然后,创建一个 `LectureRepository` 接口,继承自 `JpaRepository`,用于访问数据库:
```java
@Repository
public interface LectureRepository extends JpaRepository<Lecture, Long> {
}
```
接下来,创建一个 `AttendanceController` 类,处理签到相关的请求:
```java
@Controller
@RequestMapping("/attendance")
public class AttendanceController {
@Autowired
private LectureRepository lectureRepository;
@GetMapping("/{lectureId}")
public String showSignInPage(@PathVariable Long lectureId, Model model) {
Lecture lecture = lectureRepository.findById(lectureId).orElseThrow(() -> new RuntimeException("Lecture not found"));
model.addAttribute("lecture", lecture);
return "sign-in";
}
@PostMapping("/{lectureId}")
public String handleSignInRequest(@PathVariable Long lectureId) {
Lecture lecture = lectureRepository.findById(lectureId).orElseThrow(() -> new RuntimeException("Lecture not found"));
Attendance attendance = new Attendance();
attendance.setLecture(lecture);
attendance.setSignInTime(LocalDateTime.now());
lecture.getAttendanceSet().add(attendance);
lectureRepository.save(lecture);
return "redirect:/attendance/" + lectureId;
}
}
```
其中,`showSignInPage` 方法用于展示签到页面,`handleSignInRequest` 方法用于处理签到请求。
最后,创建一个 Thymeleaf 模板文件 `sign-in.html`,展示签到页面:
```html
<!DOCTYPE html>
<html>
<head>
<meta charset="UTF-8">
<title>医生讲座签到</title>
</head>
<body>
<h1>{{ lecture.title }}</h1>
<p>主讲人:{{ lecture.speaker }}</p>
<p>开始时间:{{ lecture.startTime }}</p>
<p>结束时间:{{ lecture.endTime }}</p>
<form method="post" action="/attendance/{{ lecture.id }}">
<input type="submit" value="签到">
</form>
</body>
</html>
```
以上就是基于 Spring Boot 实现的医生讲座签到功能的代码实现。
阅读全文
相关推荐
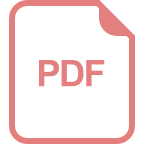
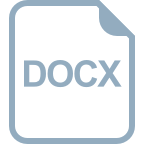
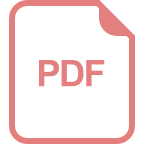
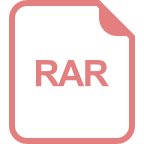
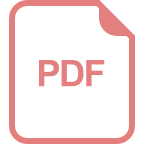
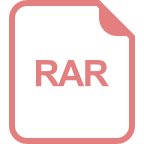
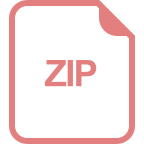
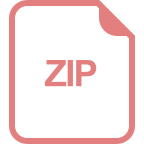
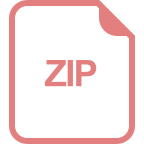
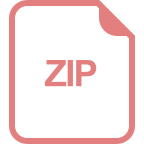
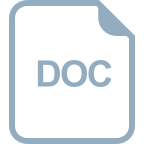
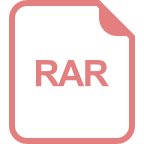
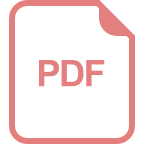
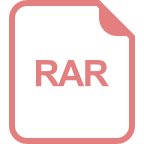
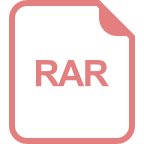
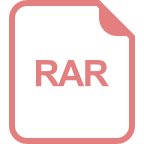
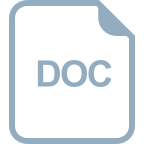
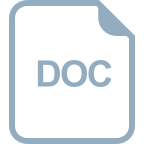