写一个ai声音合成程序
时间: 2023-09-06 19:07:33 浏览: 204
这是一个基于Python的AI声音合成程序示例,使用了Google Text-to-Speech API和pydub库。在使用之前,请确保你已经拥有Google Cloud的API密钥。
```python
import os
from google.cloud import texttospeech
from pydub import AudioSegment
# 设置Google Cloud API密钥路径
os.environ["GOOGLE_APPLICATION_CREDENTIALS"] = "path/to/api/key.json"
# Google Text-to-Speech API参数
language_code = "en-US"
voice_gender = texttospeech.enums.SsmlVoiceGender.NEUTRAL
# 创建Google Text-to-Speech客户端
client = texttospeech.TextToSpeechClient()
# 文本转语音函数
def text_to_speech(text):
# 设置声音选项
voice = texttospeech.types.VoiceSelectionParams(
language_code=language_code, ssml_gender=voice_gender
)
# 设置语音选项
audio_config = texttospeech.types.AudioConfig(
audio_encoding=texttospeech.enums.AudioEncoding.MP3
)
# 调用Google Text-to-Speech API
synthesis_input = texttospeech.types.SynthesisInput(text=text)
response = client.synthesize_speech(synthesis_input, voice, audio_config)
# 下载MP3文件
with open("output.mp3", "wb") as out:
out.write(response.audio_content)
# 合成音频文件函数
def combine_audio_files(audio_files):
combined_audio = AudioSegment.empty()
for file in audio_files:
combined_audio += AudioSegment.from_file(file)
combined_audio.export("combined_audio.mp3", format="mp3")
# 主程序
if __name__ == "__main__":
# 输入文本
text = input("请输入文本:")
# 将文本转换为语音
text_to_speech(text)
# 合成音频文件
audio_files = ["output.mp3"]
combine_audio_files(audio_files)
# 删除临时文件
os.remove("output.mp3")
```
这个示例程序会将你输入的文本转换为语音,并将语音保存为MP3文件。然后,它会将多个MP3文件合并成一个音频文件并保存为MP3格式。你可以根据自己的需求进行修改和优化。
阅读全文
相关推荐
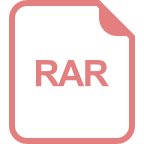
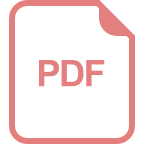
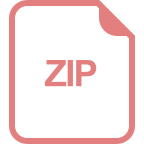
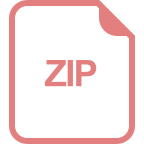
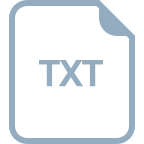
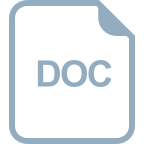
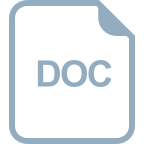
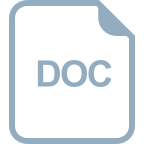
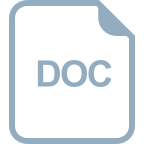
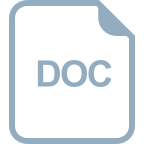
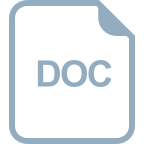
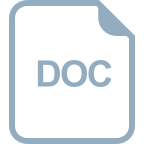
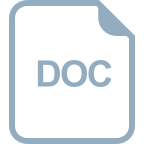
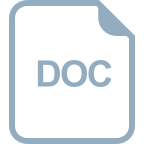
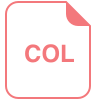
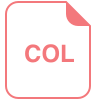
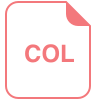