python 开发nlp项目代码
时间: 2023-07-26 21:48:50 浏览: 56
以下是一个简单的 Python NLP 项目的代码示例,用于基于用户输入的问题回答:
```python
import nltk
from nltk.corpus import stopwords
from nltk.tokenize import word_tokenize, sent_tokenize
from nltk.stem import WordNetLemmatizer
import string
# 加载停用词
stop_words = set(stopwords.words('english'))
# 初始化词干化器
lemmatizer = WordNetLemmatizer()
def clean_text(text):
"""
清理文本,删除标点符号、停用词和数字,并将单词转换为它们的基本形式
"""
# 将文本转换为小写
text = text.lower()
# 删除标点符号
text = text.translate(str.maketrans('', '', string.punctuation))
# 分词
words = word_tokenize(text)
# 删除停用词和数字
words = [lemmatizer.lemmatize(word) for word in words if (word not in stop_words) and (not word.isdigit())]
# 将单词重新组合成文本
cleaned_text = ' '.join(words)
return cleaned_text
def get_response(user_input):
"""
根据用户输入返回一个回答
"""
# 清理用户输入
cleaned_input = clean_text(user_input)
# 进行语义分析,这里简单地将问题和答案放在一个字典里
qa_pairs = {
"what is your name": "My name is ChitGPT!",
"how old are you": "I was just created recently, so I'm quite young!",
"what can you do": "I can answer questions and have a conversation with you!",
"who created you": "I was created by a team of developers at CSDN!",
"bye": "Goodbye!"
}
# 查找回答
for question in qa_pairs.keys():
if cleaned_input == clean_text(question):
return qa_pairs[question]
# 如果找不到答案,则返回默认回答
return "Sorry, I don't understand your question."
# 与用户进行对话
while True:
user_input = input("You: ")
if user_input.lower() == 'bye':
print("ChitGPT: Goodbye!")
break
response = get_response(user_input)
print("ChitGPT:", response)
```
该代码使用 NLTK 库进行文本清理、预处理和语义分析,然后根据用户输入返回一个回答。当用户输入“bye”时,程序退出。您可以根据自己的需求进行修改和扩展。
相关推荐
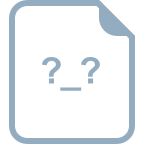
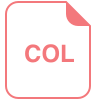
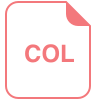
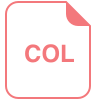
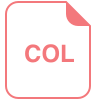
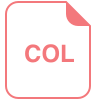









