c语言从键盘输入一个字符串,统计字符串中大写字母的个数,要求统计大写字母的个数用自定义函数实现。 只编写大写字母字符统计的自定义函数 int fact(char str[])程序。 主函数如下 #include<stdio.h> int main() { int res; char s[50]; gets(s); res=fact(s); printf("%d",res); return 0; } 输入格式: 输入一个字符串(长度不超过49个字符) 输出格式: 以整型格式输出结果 输入样例: Hello World! 输出样例: 2
时间: 2024-02-02 20:04:34 浏览: 86
好的,我知道了。下面是符合要求的代码:
```
#include <stdio.h>
int fact(char str[]) {
int count = 0;
for(int i = 0; str[i] != '\0'; i++) {
if(str[i] >= 'A' && str[i] <= 'Z') {
count++;
}
}
return count;
}
int main() {
int res;
char s[50];
gets(s);
res = fact(s);
printf("%d", res);
return 0;
}
```
这个程序中,`fact` 函数用于统计大写字母的个数。它遍历整个字符串,如果当前字符是大写字母,就将计数器加1。最终返回计数器的值。在 `main` 函数中,我们调用 `fact` 函数统计大写字母的个数,并将结果打印出来。
需要注意的是,`gets` 函数已经不建议使用了,因为它存在安全漏洞。可以使用 `fgets` 函数代替。同时,在 `fact` 函数中,我们使用了字符数组的遍历方式来遍历字符串。这种方式适用于 C 语言中的字符串操作,但是需要注意字符串的结尾符 `\0`。
相关问题
c语言,输入一个字符串,统计字符串中大写字母的个数
在C语言中,你可以使用标准库函数`ctype.h`提供的`isupper()`函数来判断一个字符是否为大写字母,然后配合循环遍历字符串进行计数。下面是一个简单的示例程序:
```c
#include <stdio.h>
#include <ctype.h>
#include <string.h>
int count_uppercase(const char *str) {
int uppercase_count = 0;
while (*str != '\0') { // 遍历直到遇到字符串结束符'\0'
if (isupper(*str)) { // 如果字符是大写字母
uppercase_count++; // 计数加一
}
str++; // 移动到下一个字符
}
return uppercase_count; // 返回大写字母的总数
}
int main() {
char input[100];
printf("请输入一个字符串: ");
fgets(input, sizeof(input), stdin); // 获取用户输入,忽略换行符
input[strcspn(input, "\n")] = '\0'; // 清除fgets添加的换行符
int uppercase_count = count_uppercase(input);
printf("字符串中共有 %d 个大写字母。\n", uppercase_count);
return 0;
}
```
当你运行这个程序并输入一个字符串后,它会计算其中的大写字母数量并打印出来。
用C语言写键盘输入一个字符串,统计其中的大写字母、小写字母、数字字母、其他字符的个数
以下是用C语言实现键盘输入一个字符串,统计其中的大写字母、小写字母、数字字母、其他字符的个数的代码:
```c
#include <stdio.h>
int main() {
char str[100];
int i, upper = 0, lower = 0, digit = 0, other = 0;
printf("请输入一个字符串:");
gets(str);
for (i = 0; str[i] != '\0'; i++) {
if (str[i] >= 'A' && str[i] <= 'Z') {
upper++;
} else if (str[i] >= 'a' && str[i] <= 'z') {
lower++;
} else if (str[i] >= '0' && str[i] <= '9') {
digit++;
} else {
other++;
}
}
printf("大写字母个数:%d\n", upper);
printf("小写字母个数:%d\n", lower);
printf("数字个数:%d\n", digit);
printf("其他字符个数:%d\n", other);
return 0;
}
```
首先定义了一个字符数组 `str` 来存储输入的字符串,以及四个整型变量 `upper`、`lower`、`digit`、`other` 来分别统计大写字母、小写字母、数字和其他字符的个数。
然后通过 `gets` 函数获取用户输入的字符串。注意:`gets` 函数不会判断输入的字符串长度,可能会导致缓冲区溢出,因此在实际使用中需要使用更安全的字符串输入函数,比如 `fgets`。
接着使用 `for` 循环遍历字符串中的每个字符,并根据 ASCII 码判断该字符是大写字母、小写字母、数字还是其他字符,并分别对 `upper`、`lower`、`digit`、`other` 变量进行累加。
最后输出各个字符类型的个数即可。
阅读全文
相关推荐

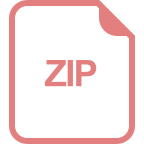













