java实现雪花算法生成8-4-4-4-12格式的UUID
时间: 2024-01-07 13:06:02 浏览: 261
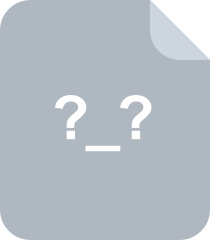
雪花算法Java代码实现的ID发号器
要在Java中使用Snowflake算法生成8-4-4-4-12格式的UUID,可以稍微修改上面的示例代码。具体来说,我们需要将48位的数字分成5个部分,其中前8位表示时间戳的高位,接下来的4位表示数据中心标识,4位表示工作机器标识,接下来的4位表示时间戳的低位,最后的36位表示序列号。
下面是一个示例代码,可用于生成8-4-4-4-12格式的UUID:
```java
import java.util.UUID;
public class SnowflakeIdGenerator {
private final long datacenterId;
private final long workerId;
private long sequence = 0L;
private final long twepoch = 1288834974657L;
private final long datacenterIdBits = 4L;
private final long workerIdBits = 4L;
private final long maxDatacenterId = -1L ^ (-1L << datacenterIdBits);
private final long maxWorkerId = -1L ^ (-1L << workerIdBits);
private final long sequenceBits = 12L;
private final long workerIdShift = sequenceBits;
private final long datacenterIdShift = sequenceBits + workerIdBits;
private final long timestampLeftShift = sequenceBits + workerIdBits + datacenterIdBits;
private final long sequenceMask = -1L ^ (-1L << sequenceBits);
private long lastTimestamp = -1L;
public SnowflakeIdGenerator(long datacenterId, long workerId) {
if (datacenterId > maxDatacenterId || datacenterId < 0) {
throw new IllegalArgumentException("datacenterId can't be greater than maxDatacenterId or less than 0");
}
if (workerId > maxWorkerId || workerId < 0) {
throw new IllegalArgumentException("workerId can't be greater than maxWorkerId or less than 0");
}
this.datacenterId = datacenterId;
this.workerId = workerId;
}
public synchronized UUID nextId() {
long timestamp = timeGen();
if (timestamp < lastTimestamp) {
throw new RuntimeException("Clock moved backwards. Refusing to generate id for " + (lastTimestamp - timestamp) + " milliseconds");
}
if (lastTimestamp == timestamp) {
sequence = (sequence + 1) & sequenceMask;
if (sequence == 0) {
timestamp = tilNextMillis(lastTimestamp);
}
} else {
sequence = 0L;
}
lastTimestamp = timestamp;
long mostSigBits = (timestamp - twepoch) << timestampLeftShift;
mostSigBits |= (datacenterId << datacenterIdShift);
mostSigBits |= (workerId << workerIdShift);
mostSigBits |= (sequence);
// Split into 5 parts
String uuidStr = String.format("%016x", mostSigBits);
String part1 = uuidStr.substring(0, 8);
String part2 = uuidStr.substring(8, 12);
String part3 = uuidStr.substring(12, 16);
String part4 = uuidStr.substring(16, 20);
String part5 = uuidStr.substring(20, 32);
// Combine the parts into UUID format
String uuid = part1 + "-" + part2 + "-" + part3 + "-" + part4 + "-" + part5;
return UUID.fromString(uuid);
}
private long tilNextMillis(long lastTimestamp) {
long timestamp = timeGen();
while (timestamp <= lastTimestamp) {
timestamp = timeGen();
}
return timestamp;
}
private long timeGen() {
return System.currentTimeMillis();
}
}
```
要使用此类生成UUID,只需实例化`SnowflakeIdGenerator`类并调用`nextId()`方法。例如:
```java
SnowflakeIdGenerator idGenerator = new SnowflakeIdGenerator(1, 1);
UUID uuid = idGenerator.nextId();
System.out.println(uuid.toString());
```
这将生成一个唯一的UUID,格式为8-4-4-4-12。在此示例中,`datacenterId`和`workerId`都设置为1,可以根据需要更改它们。
阅读全文
相关推荐
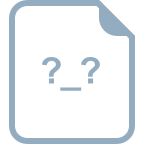

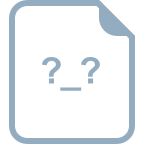
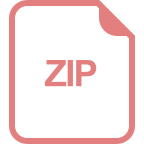
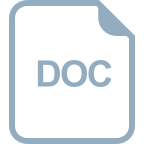
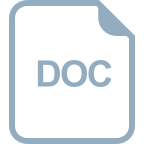
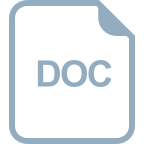







