vue d3.js 画立方体
时间: 2023-07-26 22:11:44 浏览: 94
要使用Vue和d3.js来绘制立方体,可以按照以下步骤进行:
1. 在Vue项目中安装d3.js库,可以使用npm或yarn进行安装:
```bash
npm install d3
# 或者
yarn add d3
```
2. 在Vue组件中引入d3库,并创建SVG元素:
```javascript
import * as d3 from 'd3'
export default {
mounted() {
const width = 500
const height = 500
const svg = d3.select(this.$el)
.append('svg')
.attr('width', width)
.attr('height', height)
}
}
```
3. 绘制立方体的六个面:
```javascript
const data = [
{ x: 0, y: 0, z: 0 },
{ x: 0, y: 100, z: 0 },
{ x: 100, y: 100, z: 0 },
{ x: 100, y: 0, z: 0 },
{ x: 0, y: 0, z: 100 },
{ x: 0, y: 100, z: 100 },
{ x: 100, y: 100, z: 100 },
{ x: 100, y: 0, z: 100 }
]
const faces = [
{ a: 0, b: 1, c: 2, d: 3 },
{ a: 1, b: 5, c: 6, d: 2 },
{ a: 5, b: 4, c: 7, d: 6 },
{ a: 4, b: 0, c: 3, d: 7 },
{ a: 0, b: 4, c: 5, d: 1 },
{ a: 3, b: 2, c: 6, d: 7 }
]
const polygons = svg.selectAll('polygon')
.data(faces)
.enter()
.append('polygon')
.attr('points', d => {
const points = [data[d.a], data[d.b], data[d.c], data[d.d]]
const projection = d3.geoOrthographic().rotate([0, 0, 0]).translate([width / 2, height / 2])
const path = d3.geoPath().projection(projection)
return points.map(point => {
const [x, y] = path(point)
return `${x},${y}`
}).join(' ')
})
.attr('fill', 'none')
.attr('stroke', 'black')
```
4. 通过旋转立方体来实现交互效果:
```javascript
let mouseX = 0
let mouseY = 0
svg.on('mousemove', function() {
mouseX = d3.event.offsetX
mouseY = d3.event.offsetY
})
function tick() {
const projection = d3.geoOrthographic().rotate([-mouseY / 5, -mouseX / 5, 0]).translate([width / 2, height / 2])
const path = d3.geoPath().projection(projection)
polygons.attr('points', d => {
const points = [data[d.a], data[d.b], data[d.c], data[d.d]]
return points.map(point => {
const [x, y] = path(point)
return `${x},${y}`
}).join(' ')
})
requestAnimationFrame(tick)
}
tick()
```
以上就是使用Vue和d3.js来绘制立方体的基本步骤,你可以根据需要进行调整和优化。
相关推荐
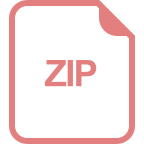
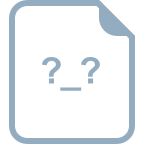
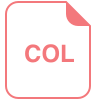
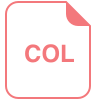
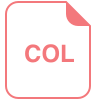
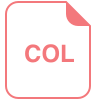
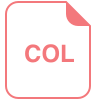









