unity中已经有Eleven的预制体,当名称为Eleven的预制体组件出现时等待1秒弹出指定弹窗代码及步骤
时间: 2024-01-21 14:18:14 浏览: 30
我们可以通过以下步骤实现:
1. 在Unity的Hierarchy视图中找到名称为Eleven的预制体,选中它并在Inspector视图中找到它的组件。
2. 添加一个名为"PopupController"的脚本组件到该预制体上。
3. 在"PopupController"脚本中编写如下代码:
```
using UnityEngine;
using System.Collections;
public class PopupController : MonoBehaviour
{
public GameObject popup; // 指定的弹窗
private void Start()
{
StartCoroutine(ShowPopup());
}
IEnumerator ShowPopup()
{
yield return new WaitForSeconds(1f); // 等待1秒
popup.SetActive(true); // 显示指定的弹窗
}
}
```
4. 将指定的弹窗拖拽到"PopupController"脚本的"popup"字段中。
5. 运行游戏并等待1秒,指定的弹窗将会弹出。
相关问题
unity中当检测名称为Eleven的预制体组件出现时等待1秒弹出指定弹窗代码及步骤
以下是实现该功能的代码和步骤:
1. 首先,在Unity中创建一个空对象,命名为“PopupManager”。
2. 在PopupManager对象上添加一个脚本,命名为“PopupTrigger”。
3. 在PopupTrigger脚本中定义一个公共GameObject变量,命名为“elevenPrefab”。
```C#
public GameObject elevenPrefab;
```
4. 在Update()方法中检测elevenPrefab是否存在,并等待1秒后弹出弹窗。
```C#
void Update()
{
if (GameObject.Find("Eleven") != null)
{
StartCoroutine(ShowPopupAfterDelay(1f));
}
}
IEnumerator ShowPopupAfterDelay(float delay)
{
yield return new WaitForSeconds(delay);
Instantiate(elevenPrefab);
}
```
5. 在Unity中创建一个弹窗UI预制体,命名为“Popup”。
6. 将Popup预制体拖拽到PopupTrigger脚本中的elevenPrefab变量中。
7. 运行游戏,当场景中出现名称为“Eleven”的预制体组件时,等待1秒后将弹出Popup预制体。
unity中当检测名称为Eleven的预制体组件出现时等待1秒弹出指定弹窗
你可以使用Unity中的协程(Coroutine)和Invoke函数来实现这个功能。
首先,在代码中检测预制体组件出现的事件,可以使用Unity的事件系统,例如OnCollisionEnter或OnTriggerEnter。在这个事件中,你可以使用协程和Invoke函数来等待1秒并弹出指定弹窗。
以下是示例代码:
```csharp
using UnityEngine;
using UnityEngine.UI;
public class Example : MonoBehaviour
{
public GameObject prefab;
public GameObject popup;
private void OnTriggerEnter(Collider other)
{
if (other.gameObject.name == "Eleven")
{
StartCoroutine(ShowPopup());
}
}
private IEnumerator ShowPopup()
{
yield return new WaitForSeconds(1f);
popup.SetActive(true);
yield return new WaitForSeconds(2f);
popup.SetActive(false);
}
}
```
在这个示例中,我们检测名为"Eleven"的预制体组件是否进入了触发器。如果是,我们就启动协程ShowPopup(),在1秒后弹出指定弹窗。弹窗显示2秒后会自动消失。
请注意,这只是一个示例代码,你需要根据自己的需求进行修改。
相关推荐
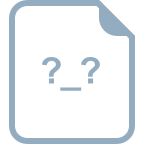












