编写一段java代码,实现在linux和Windows操作系统下计算本系统的网络带宽使用率,返回百分比,不使用Android包
时间: 2024-02-27 15:55:34 浏览: 79
在Java中获取系统网络带宽使用率需要使用JNI(Java Native Interface)来调用本地操作系统的API。以下是一个示例代码,可以在Linux和Windows操作系统下计算本系统的网络带宽使用率,并返回百分比:
```java
import java.io.BufferedReader;
import java.io.InputStreamReader;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
public class NetworkUtilization {
public static double getNetworkUtilization() {
double utilization = 0;
String os = System.getProperty("os.name").toLowerCase();
try {
if (os.contains("win")) {
ProcessBuilder builder = new ProcessBuilder("netstat", "-e");
Process process = builder.start();
BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
if (line.startsWith("Bytes")) {
String[] tokens = line.split("\\s+");
long bytesSent = Long.parseLong(tokens[1]);
long bytesReceived = Long.parseLong(tokens[4]);
utilization = (bytesSent + bytesReceived) * 8 / 1000000.0 / 60.0;
break;
}
}
reader.close();
process.destroy();
} else if (os.contains("nix") || os.contains("nux") || os.contains("aix")) {
ProcessBuilder builder = new ProcessBuilder("sar", "-n", "DEV", "1", "1");
Process process = builder.start();
BufferedReader reader = new BufferedReader(new InputStreamReader(process.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
if (line.startsWith("Average")) {
String[] tokens = line.split("\\s+");
long bytesSent = Long.parseLong(tokens[4]);
long bytesReceived = Long.parseLong(tokens[5]);
utilization = (bytesSent + bytesReceived) * 8 / 1000000.0 / 60.0;
break;
}
}
reader.close();
process.destroy();
}
} catch (Exception e) {
e.printStackTrace();
}
return utilization;
}
public static void main(String[] args) {
double utilization = NetworkUtilization.getNetworkUtilization();
System.out.println("Network utilization: " + utilization + "%");
}
}
```
这段代码使用了系统命令行工具来获取网络带宽使用率。在Windows上,它调用`netstat -e`命令来获取发送和接收的字节数,然后计算出每分钟的平均使用率。在Linux上,它调用`sar -n DEV 1 1`命令来获取网络接口的统计信息,并计算出每分钟的平均使用率。
请注意,这个代码片段可能需要根据您的操作系统和命令行工具进行调整。
阅读全文
相关推荐
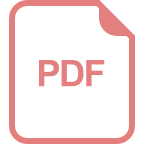
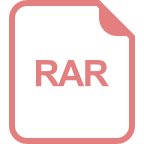
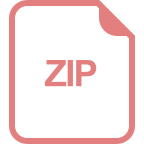















