mybatis generator 如何使用提供的example类,完成 a = 1 and (b = 2 or c =2)的逻辑
时间: 2023-06-02 12:03:52 浏览: 119
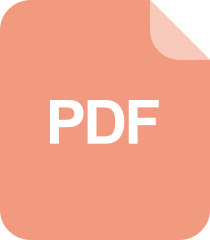
Java中mybatis关于example类的使用详解
对于这个问题,我可以给出以下回答:
使用Mybatis Generator提供的Example类可以实现查询条件拼装,其中and、or的条件可以通过Example类自带的Criteria对象实现,具体的代码实现如下:
```java
Example example = new Example(MyTable.class);
Criteria criteria = example.createCriteria();
criteria.andEqualTo("a", 1).andEqualTo("b", 2).orEqualTo("c", 2);
```
在上面的代码中,我们首先创建了一个MyTable类的Example对象,然后通过createCriteria()方法创建了一个Criteria对象,并且通过andEqualTo()和orEqualTo()方法实现了查询条件的拼装。最终的查询条件为:a=1 and (b=2 or c=2)。
通过这种方式,我们可以使用Mybatis Generator提供的Example类很方便地实现复杂的查询条件拼装。
阅读全文
相关推荐
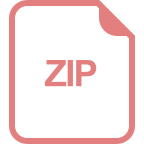
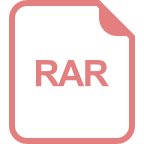

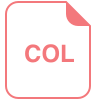
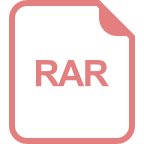
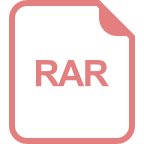
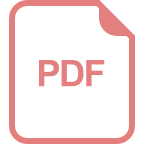
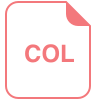
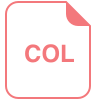
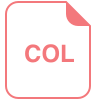
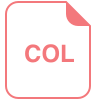

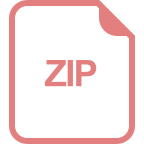
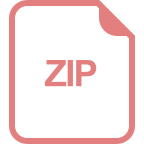
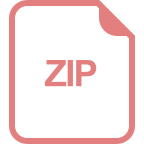
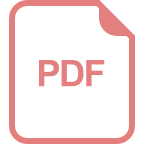
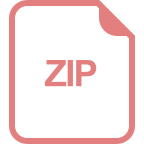
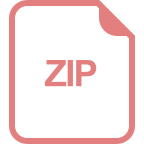
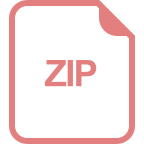