使用c++和windows api函数,实现冒泡排序的并行算法
时间: 2024-03-18 21:41:17 浏览: 79
为了实现并行算法,我们可以使用Windows API函数创建多个线程来同时执行冒泡排序。具体步骤如下:
1. 定义一个线程函数,用于执行冒泡排序。该函数接受一个整数数组和数组长度作为参数。
```cpp
DWORD WINAPI BubbleSort(LPVOID param)
{
int* arr = (int*)param;
int n = sizeof(arr) / sizeof(int);
for (int i = 0; i < n - 1; i++)
{
for (int j = 0; j < n - i - 1; j++)
{
if (arr[j] > arr[j + 1])
{
int temp = arr[j];
arr[j] = arr[j + 1];
arr[j + 1] = temp;
}
}
}
return 0;
}
```
2. 在主函数中创建多个线程,并将待排序的数组分给不同的线程处理。可以使用`CreateThread`函数创建线程,并使用`WaitForMultipleObjects`函数等待所有线程完成。
```cpp
int main()
{
const int n = 10;
int arr[n] = { 9, 8, 7, 6, 5, 4, 3, 2, 1, 0 };
HANDLE threads[2];
int subArrSize = n / 2;
threads[0] = CreateThread(NULL, 0, BubbleSort, arr, subArrSize, NULL);
threads[1] = CreateThread(NULL, 0, BubbleSort, arr + subArrSize, subArrSize, NULL);
WaitForMultipleObjects(2, threads, TRUE, INFINITE);
// 输出排序结果
for (int i = 0; i < n; i++)
{
std::cout << arr[i] << " ";
}
std::cout << std::endl;
return 0;
}
```
在上面的代码中,我们将数组分成两个子数组,每个子数组由一个线程进行排序。在排序完成后,我们使用`WaitForMultipleObjects`函数等待两个线程都完成后再输出排序结果。
需要注意的是,如果数组长度不能被线程数整除,则需要对剩余的部分进行单线程排序。此外,为了避免多个线程同时访问同一个数组元素,需要对访问数组的代码进行适当的同步。
阅读全文
相关推荐
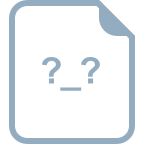
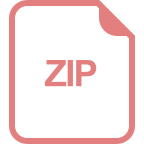

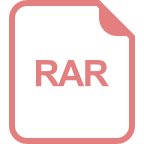
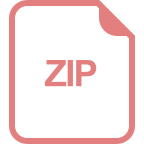
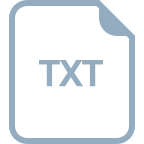
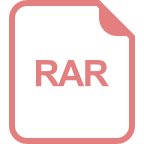
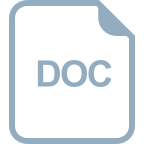
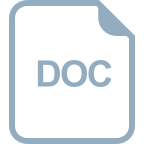
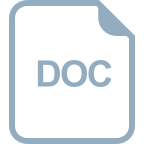
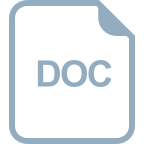
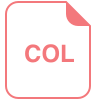
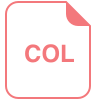
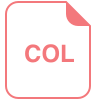
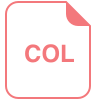
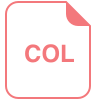
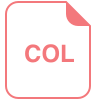