java走迷宫课程设计
时间: 2023-10-14 13:06:34 浏览: 70
以下是一个简单的 Java 迷宫游戏的课程设计:
1. 首先,你需要设计迷宫地图。可以用数组表示,其中 0 表示空格,1 表示障碍物,2 表示起点,3 表示终点。
2. 接下来,你需要编写一个类来表示迷宫游戏的状态。这个类应该包括当前位置、移动方向、已经走过的路径等信息。
3. 然后,你需要编写一个类来实现游戏逻辑。这个类应该包括游戏开始、结束、移动等方法。在移动方法中,你需要判断当前位置是否为终点,如果是则游戏结束;否则,你需要检查当前位置是否可以移动,如果可以则更新状态并继续游戏。
4. 最后,你可以编写一个简单的 GUI 来展示迷宫地图和游戏状态。你可以用 JLabel 表示地图,用 JTextArea 显示游戏状态,用 JButton 实现移动操作。
下面是一个简单的迷宫游戏的代码示例,供你参考:
```java
public class MazeGame {
private int[][] map;
private int startX, startY;
private int endX, endY;
private int curX, curY;
private int direction;
private List<Integer[]> path;
public MazeGame(int[][] map, int startX, int startY, int endX, int endY) {
this.map = map;
this.startX = startX;
this.startY = startY;
this.endX = endX;
this.endY = endY;
curX = startX;
curY = startY;
direction = 0;
path = new ArrayList<>();
path.add(new Integer[]{curX, curY});
}
public boolean move() {
int nextX = curX, nextY = curY;
switch (direction) {
case 0:
nextY--;
break;
case 1:
nextX++;
break;
case 2:
nextY++;
break;
case 3:
nextX--;
break;
}
if (nextX == endX && nextY == endY) {
path.add(new Integer[]{nextX, nextY});
return true;
}
if (map[nextX][nextY] == 0) {
curX = nextX;
curY = nextY;
path.add(new Integer[]{curX, curY});
return false;
}
direction = (direction + 1) % 4;
return false;
}
public List<Integer[]> getPath() {
return path;
}
}
```
```java
public class MazePanel extends JPanel {
private int[][] map;
private MazeGame game;
private JLabel[][] cells;
public MazePanel(int[][] map) {
this.map = map;
game = new MazeGame(map, 1, 1, map.length - 2, map[0].length - 2);
int rows = map.length;
int cols = map[0].length;
setLayout(new GridLayout(rows, cols));
cells = new JLabel[rows][cols];
for (int i = 0; i < rows; i++) {
for (int j = 0; j < cols; j++) {
cells[i][j] = new JLabel();
if (map[i][j] == 1) {
cells[i][j].setBackground(Color.BLACK);
} else {
cells[i][j].setBackground(Color.WHITE);
}
add(cells[i][j]);
}
}
cells[1][1].setBackground(Color.GREEN);
cells[rows - 2][cols - 2].setBackground(Color.RED);
Timer timer = new Timer(500, new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
if (game.move()) {
((Timer) e.getSource()).stop();
for (Integer[] p : game.getPath()) {
cells[p[0]][p[1]].setBackground(Color.YELLOW);
}
} else {
Integer[] p = game.getPath().get(game.getPath().size() - 2);
cells[p[0]][p[1]].setBackground(Color.WHITE);
p = game.getPath().get(game.getPath().size() - 1);
cells[p[0]][p[1]].setBackground(Color.GREEN);
}
}
});
timer.start();
}
}
```
```java
public class MazeFrame extends JFrame {
public MazeFrame(int[][] map) {
setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
setSize(500, 500);
MazePanel panel = new MazePanel(map);
setContentPane(panel);
setVisible(true);
}
public static void main(String[] args) {
int[][] map = {
{1, 1, 1, 1, 1, 1, 1},
{1, 0, 0, 0, 0, 0, 1},
{1, 0, 1, 1, 1, 0, 1},
{1, 0, 1, 0, 0, 0, 1},
{1, 0, 0, 0, 1, 0, 1},
{1, 0, 1, 0, 0, 0, 1},
{1, 1, 1, 1, 1, 1, 1}
};
new MazeFrame(map);
}
}
```
在这个例子中,我们使用了一个 JPanel 来展示地图和游戏状态。在地图上,我们用 JLabel 表示每个单元格,并用不同的颜色表示不同的状态。在游戏逻辑中,我们使用一个 MazeGame 类来表示游戏状态,其中包括当前位置、移动方向、已经走过的路径等信息。在移动方法中,我们检查当前位置是否为终点,如果是则游戏结束;否则,我们检查当前位置是否可以移动,如果可以则更新状态并继续游戏。在 GUI 中,我们使用一个 Timer 来控制游戏的进程,每次调用 MazeGame 的 move 方法来移动角色,并更新地图状态。最后,当游戏结束时,我们用黄色的颜色表示已经走过的路径。
相关推荐
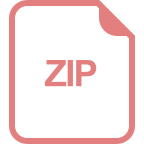
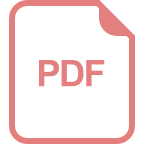
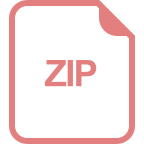
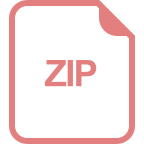
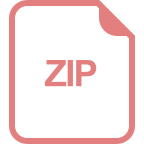
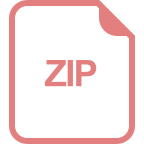
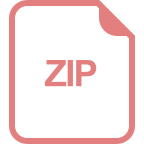
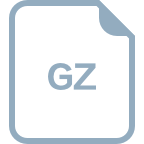
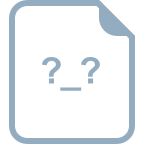
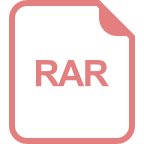
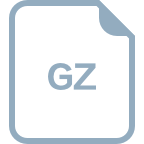