#include <stdio.h> #include <string.h> void escape(char *s, char *t) { int i = 0, j = 0; while (t[i] != '\0') { if (t[i] == '\n') { s[j++] = '\\'; s[j++] = 'n'; } else if (t[i] == '\t') { s[j++] = '\\'; s[j++] = 't'; } else { s[j++] = t[i]; } i++; } s[j] = '\0'; } int main() { char t[1000], s[2000]; fgets(t, sizeof(t), stdin); escape(s, t); printf("%s", s); return 0; }这个代码怎么修改才能使它不会输出\n就结束
时间: 2024-01-16 16:05:47 浏览: 26
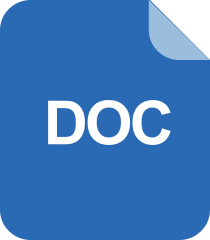
#include "stdio.h" /* 输入输出函数的头文件 */
这段代码是实现将输入的字符串中的特殊字符(如换行符、制表符)转义为可见字符(如\n、\t)。输出中包含\n是因为在C语言中,printf函数会自动在输出的末尾添加一个换行符。
如果你不想让程序在输出时自动添加换行符,只需将printf函数的格式控制字符串中的\n删除即可。下面是修改后的代码:
```c
#include <stdio.h>
#include <string.h>
void escape(char *s, char *t) {
int i = 0, j = 0;
while (t[i] != '\0') {
if (t[i] == '\n') {
s[j++] = '\\';
s[j++] = 'n';
} else if (t[i] == '\t') {
s[j++] = '\\';
s[j++] = 't';
} else {
s[j++] = t[i];
}
i++;
}
s[j] = '\0';
}
int main() {
char t[1000], s[2000];
fgets(t, sizeof(t), stdin);
escape(s, t);
printf("%s", s); // 去掉\n
return 0;
}
```
阅读全文
相关推荐
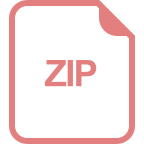
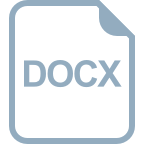






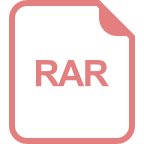
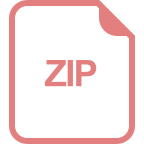
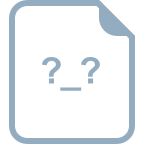
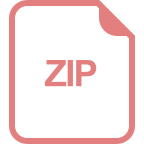
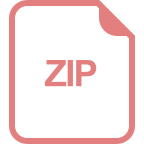
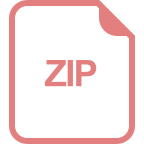