编译原理词法分析实验
时间: 2023-07-20 11:06:14 浏览: 49
词法分析是编译原理中的重要内容,其作用是将源程序中的字符序列转换成单词序列。在词法分析中,我们需要使用正则表达式来描述单词的形式,然后根据这些正则表达式来生成有限状态自动机(DFA),最后使用该自动机来识别源程序中的单词。
下面是一个简单的词法分析实验,我们将使用 C++ 语言来实现。
假设我们需要识别的单词有以下几种:
- 整型常量:由数字组成的字符串,如 123、456 等。
- 标识符:由字母、数字和下划线组成的字符串,且第一个字符必须是字母,如 a、b、c、x1、y2 等。
- 运算符:包括加、减、乘、除和取余等运算符,如 +、-、*、/、% 等。
- 分隔符:包括逗号、分号、括号和花括号等,如 ,、;、(、){、} 等。
我们可以先定义一个 Token 类,用于表示一个单词:
```cpp
class Token {
public:
enum Type {
INTEGER, IDENTIFIER, OPERATOR, SEPARATOR
};
Token(Type type, const std::string& lexeme) : type_(type), lexeme_(lexeme) {}
Type type() const { return type_; }
const std::string& lexeme() const { return lexeme_; }
private:
Type type_;
std::string lexeme_;
};
```
然后定义一个词法分析器类 Lexer:
```cpp
class Lexer {
public:
Lexer(const std::string& input) : input_(input), pos_(0) {}
Token next_token() {
while (pos_ < input_.size()) {
if (std::isdigit(input_[pos_])) {
return lex_integer();
} else if (std::isalpha(input_[pos_])) {
return lex_identifier();
} else if (is_operator(input_[pos_])) {
return lex_operator();
} else if (is_separator(input_[pos_])) {
return lex_separator();
} else {
pos_++;
}
}
return Token(Token::SEPARATOR, "");
}
private:
std::string input_;
std::size_t pos_;
bool is_operator(char c) const {
return c == '+' || c == '-' || c == '*' || c == '/' || c == '%';
}
bool is_separator(char c) const {
return c == ',' || c == ';' || c == '(' || c == ')' || c == '{' || c == '}';
}
Token lex_integer() {
std::string lexeme;
while (pos_ < input_.size() && std::isdigit(input_[pos_])) {
lexeme += input_[pos_];
pos_++;
}
return Token(Token::INTEGER, lexeme);
}
Token lex_identifier() {
std::string lexeme;
while (pos_ < input_.size() && (std::isalnum(input_[pos_]) || input_[pos_] == '_')) {
lexeme += input_[pos_];
pos_++;
}
return Token(Token::IDENTIFIER, lexeme);
}
Token lex_operator() {
std::string lexeme;
lexeme += input_[pos_];
pos_++;
return Token(Token::OPERATOR, lexeme);
}
Token lex_separator() {
std::string lexeme;
lexeme += input_[pos_];
pos_++;
return Token(Token::SEPARATOR, lexeme);
}
};
```
在 Lexer 类中,我们定义了一个私有函数来判断一个字符是否是运算符或分隔符。然后我们分别实现了 lex_integer()、lex_identifier()、lex_operator() 和 lex_separator() 函数来识别对应的单词。这些函数都会返回一个 Token 对象。
最后,我们可以在 main() 函数中使用 Lexer 类:
```cpp
int main() {
std::string input = "a = 123 + 456;";
Lexer lexer(input);
Token token;
while ((token = lexer.next_token()).type() != Token::SEPARATOR) {
std::cout << "type: " << token.type() << ", lexeme: " << token.lexeme() << std::endl;
}
return 0;
}
```
输出结果为:
```
type: IDENTIFIER, lexeme: a
type: OPERATOR, lexeme: =
type: INTEGER, lexeme: 123
type: OPERATOR, lexeme: +
type: INTEGER, lexeme: 456
type: SEPARATOR, lexeme: ;
```
这就是一个简单的词法分析器实验。实际上,在实际编译器中,词法分析器的实现要比这个复杂得多。但是,这个实验可以帮助你更好地理解词法分析的原理和实现方式。
相关推荐
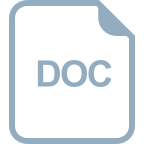













