pyqt5在tableWidget中显示excel数据
时间: 2024-05-11 20:16:12 浏览: 171
可以通过使用Python的pandas库来实现在PyQt5的tableWidget中显示Excel数据。具体步骤如下:
1.安装必要的库:
```
pip install pandas openpyxl PyQt5
```
2.导入必要的库:
```python
import pandas as pd
from PyQt5.QtWidgets import QTableWidget, QTableWidgetItem
```
3.读取Excel文件:
```python
df = pd.read_excel('your_file_path.xlsx', index_col=None, header=None)
```
4.将DataFrame中的数据逐行添加到tableWidget中:
```python
for row in range(df.shape[0]):
for column in range(df.shape[1]):
item = QTableWidgetItem(str(df.iloc[row, column]))
tableWidget.setItem(row, column, item)
```
5.最终代码示例:
```python
import pandas as pd
from PyQt5.QtWidgets import QApplication, QTableWidget, QTableWidgetItem
class ExcelTable(QTableWidget):
def __init__(self, df):
super().__init__(df.shape[0], df.shape[1])
for row in range(df.shape[0]):
for column in range(df.shape[1]):
item = QTableWidgetItem(str(df.iloc[row, column]))
self.setItem(row, column, item)
if __name__ == '__main__':
app = QApplication([])
df = pd.read_excel('your_file_path.xlsx', index_col=None, header=None)
tableWidget = ExcelTable(df)
tableWidget.show()
app.exit(app.exec_())
```
阅读全文
相关推荐
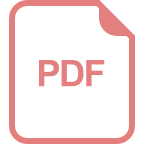
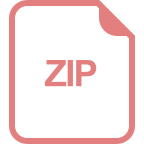
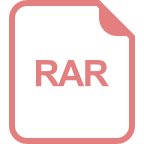
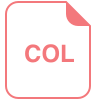





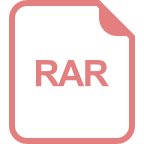
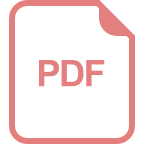
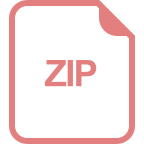
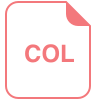





